Mastering PHP Unit Testing: A Complete Overview
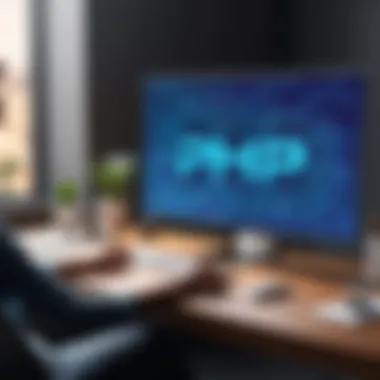
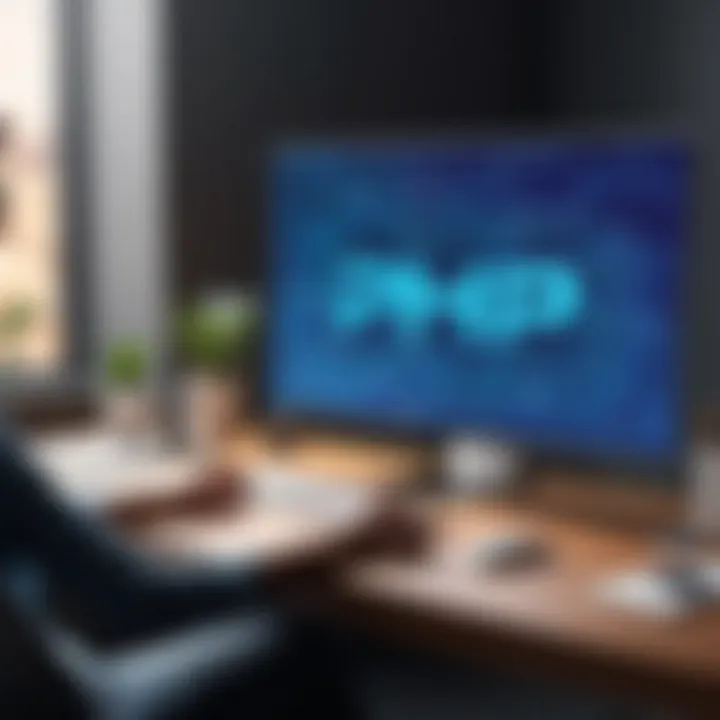
Intro
Unit testing is an integral part of software development, especially in programming languages like PHP. Developers often write code without testing it immediately, leading to bugs down the line. PHP unit testing challenges this approach by prioritizing code reliability. It helps catch issues early, ensuring that every piece of code functions as intended. In this guide, we will explore the significance of PHP unit testing, offering a comprehensive understanding of methodologies, frameworks, and best practices.
Coding Challenges
Coding challenges enhance a developer’s understanding of PHP unit testing. They provide real-world scenarios where debugging and refactoring may happen. Each challenge helps establish creativity and analytic skills. Engaging with these activities can lead to greater profficiency in unit testing.
Weekly Coding Challenges
Participating in weekly coding challenges can motivate developers to improve their skills continuously. These challenges often focus on specific testing practices. For example, a week dedicated to PHPUnit showcases the importance of this primary testing framework.
Problem Solutions and Explanations
After attempting coding challenges, reviewing solutions is crucial. Information obtained in debugging practices helps solidify concepts like test-driven development (TDD) and behavior-driven development (BDD). Solutions often illustrate how unit tests mitigate common errors.
Tips and Strategies for Coding Challenges
Consider the following strategies when tackling coding challenges:
- Understand the problem clearly: Read instructions thoroughly before writing any code.
- Break down the problem: Identify smaller components to tackle.
- Write test cases: Develop tests as you go to ensure code validity.
- Review existing solutions: Look through various approaches to gain insights.
Learning through challenges fosters a deeper connection with the practical aspects of coding.
Community Participation Highlights
Engaging with a community around coding challenges broadens one’s perspective. Forums and discussion groups often share insights on intricate coding problems. Participating in groups on platforms like Reddit elevates the learning experience further, providing needed support.
Technology Trends
As PHP continues to evolve, developers must stay informed of technology trends impacting unit testing. Keeping abreast of guidelines and standards helps enhance testing methodologies currently in practice.
Latest Technological Innovations
Recent innovations emphasize automation in unit testing. Tools like Laravel’s testing framework have accelerated this process, providing steps as easy as watching code volume increase. Thus, developers gain quick fixes to their approaches.
Emerging Technologies to Watch
The rise of container technology, such as Docker, offers ways to create isolated environments. This essentially aids unit testing by preparing conditions similar to production. When development overlaps production, reliability can shift rapidly.
Technology Impact on Society
Unit testing has influenced the way software is produced globally. Reliable software results in many real-world applications across businesses and lives. Enhanced technology has made scripts essential, challenging old manual practices.
Expert Opinions and Analysis
Insights from thought leaders in the field can shine light on innovative testing practices. Analyzing these discussions could lead to discovering new possibilities and reducing the weight of strict methodologies.
Coding Resources
Having access to the right coding resources remains essential for building effective unit testing knowledge. The following resources can guide aspiring and experienced programmers alike.
Programming Language Guides
Numerous online guides are accessible for programmers eager to enhance their PHP testing skills. Websites like Wikipedia cover both introductory and advanced principles.
Tools and Software Reviews
Using the right tools improves the effectiveness of unit testing practices. Choices range from PHPUnit to Mockery. Each tool has its unique strengths tailored to different testing approaches.
Tutorials and How-To Articles
Various online tutorials provide in-depth, hands-on details regarding coding techniques. Detailed articles result in improved understandings of unit testing concepts, building a foundation for better PH code.
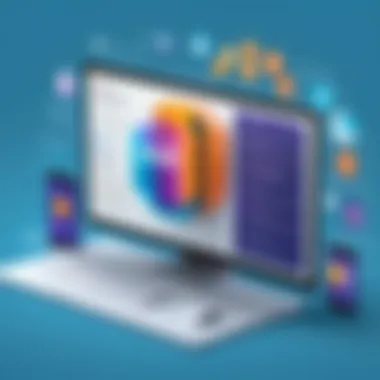
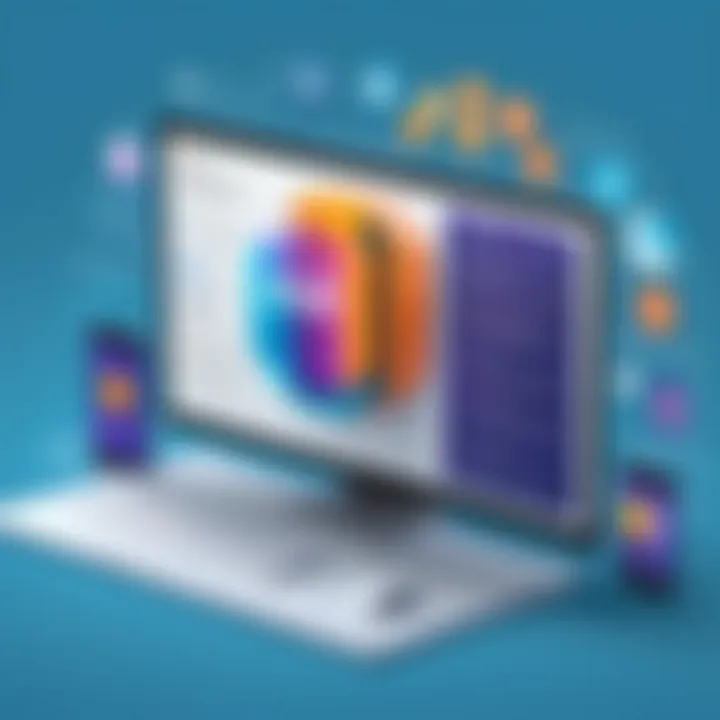
Online Learning Platforms Comparison
Education sites like Codecademy and Pluralsight facilitate learning PHP unit testing element. Evaluating these platforms can help to select the best option that suits different personal learning styles.
Computer Science Concepts
A competent understanding of computer science concepts will enrich one's capability to implement unit testing better. Familiarity with core ideals serves as a background for enhancing practical programming skills.
Algorithms and Data Structures Primers
Recognizing various algorithms accelerates debugging situations. Different structures determine results based on tests. Pulling resources to enhance this foundational knowledge is particularly valuable.
Artificial Intelligence and Machine Learning Basics
The convergence of these technologies into software development has reshaped perspective on testing methodologies. Learning how machine learning can complement unit tests is becoming important for dev leaders.
Networking and Security Fundamentals
Unit tests also touch the domain of security to ensure robustness. Familiarity with essential networking protocols reinforces better software stability.
Quantum Computing and Future Technologies
Looking to the future, understanding the prospects of quantum computing can establish unit testing into new dimensions. These technologies may lead to redefined methods where predictions meet unparalleled challenges.
Foreword to PHP Unit Testing
Unit testing stands as a cornerstone in the field of software development. For developers who work with PHP, understanding unit testing exercises a significant impact on the quality and reliability of their code. PHP unit testing aids in identifying errors before they propagate through the Software Development Life Cycle (SDLC). The central premise of unit testing is the ability to test individual components of the application in isolation. This feature not only enhances debugging efficiency but also cultivates a more organized and maintainable codebase. Here, we proceeed to couver why it maters.
What is Unit Testing?
Unit testing refers to the practice of testing the smallest sections of code, typically functions and methods, for correctness. A unit test guarantees that each piece behaves as intended. Proper unit testing involves both writing the test and running it. By focusing on these smaller pieces, developers can pinpoint where an issue originated rather than unraveling large swathes of code, easing the debugging process. When unit tests are integrated from the outset, they set expectations for each unit's behavior, offering reliable targets for future code changes.
Unit tests are automated, increasing efficiency. Automated testing frameworks assist in running these tests whenever the code is modified. As a result, they promote quick mitigation of potential errors, ensuring more robust software readiness before deployment.
The Importance of Unit Testing in PHP
The advantages of unit testing are numerous. Generally, they improve code quality in several key ways. Performing unit tests continuously offers immediate feedback. This gradual testing eliminates bugs before they escalate into massive issues. Moreover, unit tests act as documentation for the system. When engineers review the tests, they can better understand the functional scope of components. They illustrate how various parts interrelate as well, providing insights during maintenance.
Furthermore, strong unit testing practices correlate significantly with the principles of agile development, allowing for faster amendments without compromising quality. Agile methodologies advocate iterative developments and unit tests become the safety net that enables experimentation with minimal risk.
Lastly, unit testing improves collaboration across various roles in tech. When developers produce or refine code, they are more assured of its operability when unit tests are present. These tests also provide a quieting assurance when different teams work on the same codebase. All of these benefits accumulate, resulting in timely releases of higher quality PHP applications. Unit testing propels developers toward not just accomplishing tasks efficiently but also contributes to fostering a culture of testing and quality within the organization.
Overview of PHP Testing Frameworks
In the landscape of software developement, testing frameworks serve as vital tools. They offer a structured environment for validating code. PHP, a prominent programming language, features several testing frameworks. Understanding the available options can significantly enhance the efficiency and quality of unit tests.
Choosing the right framework influences not only the testing process but also the overall quality of the software. Developers must consider factors such as ease of integration, community support, and documentation when selecting a framework. This exploration is crucial for both new and experienced programmers alike.
PHPUnit: The Leading Framework
PHPUnit is the standard testing framework for PHP. Its prominence comes from its rich features and support within the PHP community. Developers often praise its straightforward installation process and comprehensive documentation which facilitate adoption.
Installation and Setup
Installing PHPUnit is a relatively simple process. The recommended method involves using Composer, a dependency manager specifically for PHP. With a few commands, users can integrate PHPUnit into their projects swiftly. This ease of installation is a significant factor in its continued popularity.
The unique aspect of PHPUnit’s setup lies in its compatibility with different PHP versions. This ensures that even legacy projects can benefit from modern testing practices. Many teams find it integrates smoothly with continuous integration environments, further making it a preferred choice.
Basic Usage
Once PHPUnit is installed, utilizing it becomes straightforward. The basic usage involves writing test cases that extend the class. This class provides essential methods like and to validate behavior.
Its simplicity ensures that programmers can quickly write and execute tests. Additionally, PHPUnit offers flexible configuration options, allowing teams to tailor it to their workflows. At the same time, new users might face challenges learning the full spectrum of PHPUnit’s capabilities. Thus, a thorough understanding is essential for maximizing its potential.
Alternative Testing Frameworks
While PHPUnit is widely accepted, other frameworks also hold value in specific contexts. They provide different perspectives on testing in PHP and may suit particular projects better.
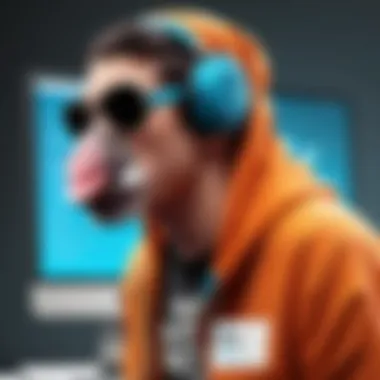
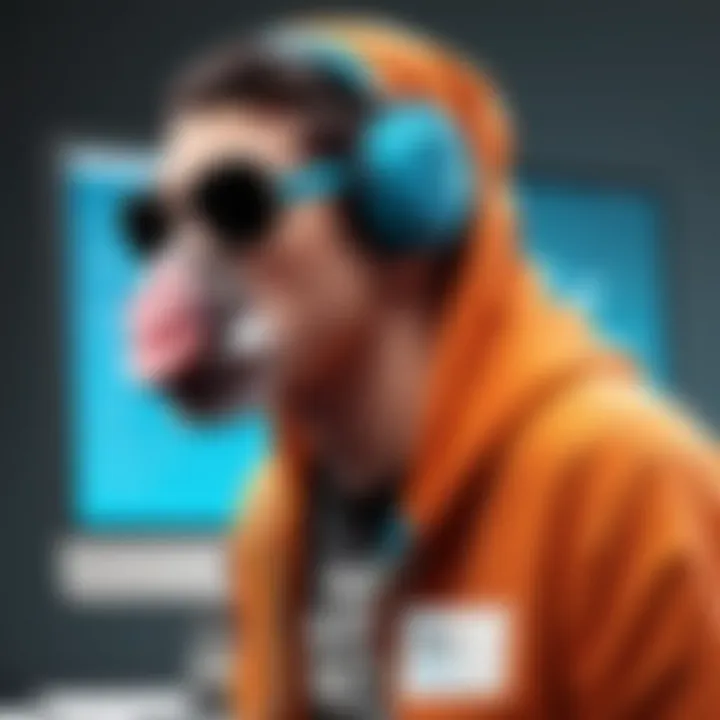
Codeception
Codeception stands out by integrating multiple testing approaches. Unlike more traditional frameworks, it combines unit, functional, and acceptance tests within a single mechanism. This versatility allows teams to address various testing needs without switching tools.
The key characteristic of Codeception is its focus on behavior-driven development (BDD). This methodology can simplify the writing of tests as it encourages using a language closely resembling human speech. The ease of articulating scenarios makes Codeception attractive for non-technical stakeholders in the project, enhancing overall communication.
SimpleTest
SimpleTest, while less commonly used than PHPUnit, offers a minimalist approach that appeals to certain developers. It is lightweight and straightforward. The principle behind SimpleTest is to enable a simpler entry point into unit testing.
A core advantage of SimpleTest is its clear documentation and ease of understanding. Beginners often find it less intimidating than larger frameworks. However, SimpleTest may lack some advanced features of competitors, which could limit its efficacy in complex projects.
These testing frameworks exhibit distinct characteristics, creating a range of options for PHP development teams. Each has advantages and suitable scenarios depending on a team's specific needs.
Writing Effective Unit Tests
Writing effective unit tests is crucial in ensuring that the code behaves as intended. This process minimizes bugs and enhances code reliability. Effective tests lead to better feedback for developers, as flawed code is caught earlier in the development cycle. A deeply structured approach significantly reduces overall project costs and enhances team collaboration.
Test Structure and Organization
Naming Conventions
Naming conventions are vital for writing unit tests that are self-explanatory and easy to navigate. When tests are well-named, clarity is achieved. Therefore, they tell a story of what they are testing. A clear name usually begins with the unit being tested, followed by the action and the expected output, such as . This provides an immediate understanding of what the test is about.
An advantage is that it aids in quick comprehension when working in teams or on legacy code. Consistency in naming also means reduced onboarding time for new developers. That said, focusing too narrowly on naming may lead to verbosity, overwhelming the reader.
Folder Structure
A carefully organized folder structure helps in maintaining test cases. Keeping tests categorized logically makes retrieval easier. For instance, class-based folder organization reflects the structure of the production code, with named folders like containing tests for the class.
This kind of arrangement provides clarity and makes it easier to locate specific tests. However, attention to maintainability is necessary because over-categorization could lead to difficulty in tracing tests. Well-thought-out structures tend to aid the development workflow, fostering better integration of unit tests in the continuous integration systems.
Techniques for Writing Tests
Arrange-Act-Assert Pattern
The Arrange-Act-Assert (AAA) pattern categorizes test-writing. It is a straightforward way of organizing tests into three clear sections: setting up (Arrange), triggering the process (Act), and asserting the expected outcomes (Assert). This clear demarcation focuses on readability, making tests intuitive and systematic.
Using this pattern could also enhance collaboration among testers and developers. It streamlines discussions and documentation since developers can quickly identify how tests build upon each other. However, some might find it somewhat limiting if overly adhered to in more heterogeneous testing environments.
Mocking Dependencies
Mocking dependencies allows for testing components in isolation. It involves creating pretend versions of complex objects your unit may depend on. By mocking, you can limit side effects, thereby focusing on testing specific functionality. This greatly enhances accuracy and control over the tests.
Utilizing mocking not only simplifies it enables clever and faster test executions. Care should be taken, however, as over-mocking can lead to false confidence in tests. Mocking can dilute the strictly relevant interactions with dependencies, possibly breaking the closer reflection between tests and actual production code responses.
Running PHP Unit Tests
Running PHP unit tests is a critical phase in the software development lifecycle. Effective execution of unit tests ensures that your code behaves as expected, detects defects early, and helps maintain quality over time. Learning how to run your checks efficiently can vastly improve the robustness of any PHP application.
Command Line Execution
Command line execution of PHP unit tests is a straightforward method. It grants developers a precise way to execute tests without the need for additional interfaces or tools. PHPUnit, the main testing framework for PHP, is typically utilized for this purpose.
To run tests from the command line, you simply open your terminal. Navigate to the directory where your test files reside and run PHPUnit with a command like this:
This command will scan the tests/ folder for test classes and execute them. By reviewing command line feedback, you gain immediate insights into which tests passed or failed. Understanding the output can be beneficial; PHPUnit provides a clear breakdown, showing durations and detailed messages for failed tests. This swift feedback loop enables instant iteration and refinement of your code and tests.
Integrating with / Pipelines
Integrating unit tests within CI/CD pipelines, like Jenkins or GitHub Actions, has transformative potential in software development. It automates the testing process and ensures that tests are consistently run with every change to the codebase, dramatically increasing reliability and reducing the likelihood of bugs arriving in production.
Jenkins Integration
Jenkins can significantly facilitate PHP unit testing. Its key characteristic is that it is an open source automation server, which allows continuous integration processes to be set up effortlessly. Once configured, Jenkins can monitor repository changes. When a change occurs, Jenkins can automatically trigger a test suite execution.
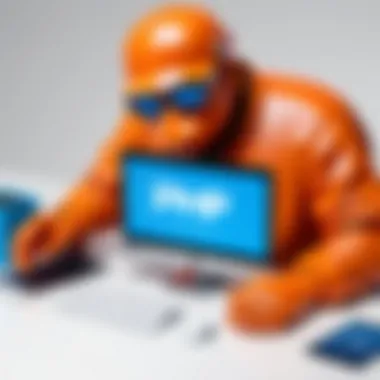
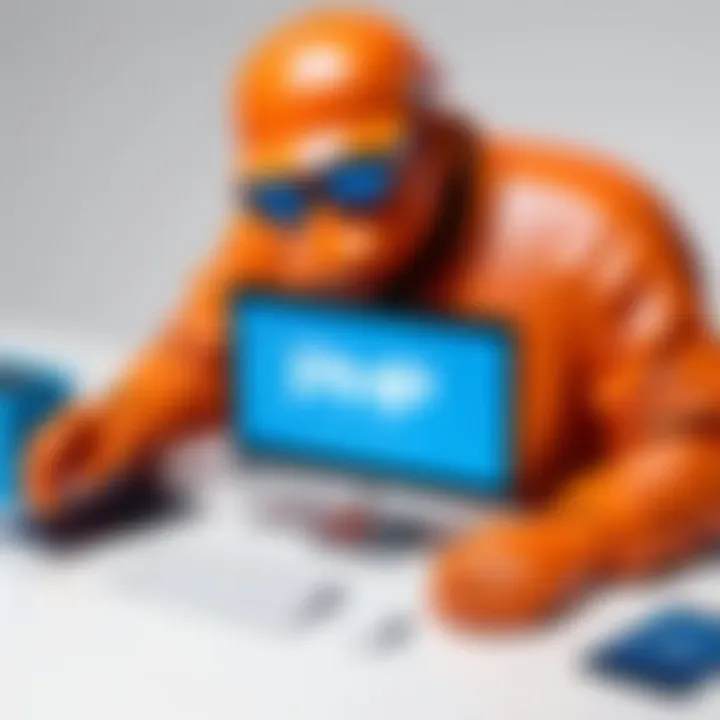
One unique feature of Jenkins is its ability to handle a multitude of plugins, broadening its integration capacity with various systems. This flexibility makes it possible to customize test configurations and reports extensively. Benefits of using Jenkins include time savings and systematic error reduction in deployments; but there are downsides, such as setup complexity that may challenge new users initially.
GitHub Actions
GitHub Actions represent another powerful tool for integrating PHP unit testing within a CI/CD setup. A key characteristic of GitHub Actions is that it is seamlessly part of the GitHub ecosystem, making it easy to automate workflows directly linked to development processes.
The unique feature of GitHub Actions lies in its flexibility and simplicity. Users can define workflows in simple YAML files, establishing clear pipelines for testing applications. The benefits here include real-time feedback and ease of use for teams already using GitHub. However, as with any tool, there are considerations regarding limitation on lengthy executions or sophisticated scenarios, which should be evaluated per project needs.
Committing to running PHP unit tests consistently safeguards code quality through proactive measures.
Common Challenges and Solutions
Understanding the common challenges encountered during PHP unit testing is crucial for effective test management. Below are key elements that programmers face in this process. Identifying challenges in advance can guide developers in implementing practical solutions, leading to smoother development cycles and better software quality.
Dealing with Legacy Code
Legacy code presents a significant barrier when trying to implement unit tests. This code is often outdated, poorly documented, and might not have been designed with testing in mind. Many times, legacy systems require an understanding of old design paradigms, which complicates the path to writing effective tests. Here are some strategies to address these issues:
- Incremental Refactoring: Gradually refactor legacy code to make it more testable. Target small sections methodically rather than attempting a large overhaul at once.
- Use of Wrapper Functions: Create wrapper functions for less modular code sections. This can allow for easier testing by isolating functionalities while minimizing disruptions to other parts of the system.
- Write Integration Tests First: Understand current behavior before implementing unit tests. Using integration tests can uncover how components interact before unit tests are created.
Adopting these strategies can aid in making legacy codebases more amenable to unit testing. The effort spent in making changes will ultimately enhance not just testability but also maintainability.
Handling Flaky Tests
Flaky tests are tests that sometimes fail and sometimes pass without changes in the underlying code. They create confusion and can undermine trust in automated testing. Investigating flaky tests requires patience to identify the root causes. Some regular culprits include:
- Environmental Dependencies: Tests often fail due to environmental factors rather than code. Factors like filesystem access, network requests, or databases can yield intermittent results.
- Shared Stateful Resources: When tests rely on shared resources, it can lead to unpredictable outcomes. Isolating each test and removing shared state can mitigate this issue.
- Timing Issues: Tests may be affected by race conditions or timing attributes. Making tests deterministic helps reduce flakiness. This can include using timeouts and mock tests for asynchronous processes.
To address flaky tests:
- Stabilize Fixtures and Environments: Ensure that the tests are run in a consistent environment to reduce variations in test outcomes.
- Regular Checkout and Review: Having regular code review sessions can help identify broader issues that could contribute to flakiness, ensuring consistency in tests.
A focus on remedying flaky tests can significantly improve the reliability of the overall testing suite. Stability is essential for any team relying on testing frameworks to maintain and enhance code quality overall.
Key Takeaway: Address challenges such as legacy code and flaky tests systematically, as they impede progress in unit testing. Addressing these challenges can lead to better software quality and enhanced trust in automated tests.
Best Practices for PHP Unit Testing
Unit testing is a foundational practice in software development that directly relates to overall code quality and reliability. Best practices for PHP unit testing are essential in creating tests that are not only effective but maintainable and easy to read. Adhering to best practices ensures that tests can evolve alongside the code base without becoming a bottleneck.
Implementing best practices can yield numerous benefits. To begin with, they lead to better maintainability of code. When tests are structured wisely, they reduce the effort required to decipher, modify, or extend tests during refactoring. Furthermore, good practices elevate code readability, making it easier for team members to understand complex logic within unit tests. This helps new team members onboard quickly and reduces the likelihood of misunderstandings that could lead to bugs.
Writing Maintainable Tests
To write maintainable tests, simplicity is key. Following the Arrange-Act-Assert pattern provides a clear way to structure tests. It separates the preparation, execution, and validation steps of testing, making it easy for programmers to follow the intent behind each test.
Use descriptive names for your test functions. Clear names like signal clearly what behavior the test is validating.
Additionally, keep in mind the DRY principle, which stands for
The Future of PHP Unit Testing
The significance of understanding the future of PHP unit testing cannot be understated. As technology progresses at an accelerated rate, the methods and standards for testing applications evolve as well. Adapting to these changes is essential for developers aiming to create reliable and maintainable code. The future landscape of PHP unit testing will be influenced by several critical elements, which enhances the overall quality of software applications.
Emerging Trends in Testing
The landscape of PHP unit testing is changing. Emerging trends drive innovation in testing processes. One pertinent trend is the increase of Behavior Driven Development (BDD). This methodology focuses on the behavior of software before it's built, aligning code development closely with expected outcomes. Therefore, frameworks such as Behat and PHPSpec are becoming more relevant. They assist teams in understanding requirements and facilitate smoother collaboration between developer, testers, and business analysts.
Another trend to observe is the integration of artificial intelligence in testing. Intelligent tools can analyze past test failures and suggest solutions to improve code. Automation is enhancing speed and accuracy as more testers relied on script-based testing.
Lastly, continuous testing is gaining traction. The necessity for agile methodologies means constant testing. This strategy supports swift feedback loops to minimize defects early in the lifecycle, which is in line with Continuous Integration/Continuous Deployment practices.
Evolving Standards in Software Testing
The evolution of standards in software testing closely ties with the paradigm shift towards quality as a focus point rather than just a checklist. New frameworks often come with best practices that redefine what it means to have a solid test suite. Testing frameworks are now focusing on code readability and simplicity, making it easier for teams to maintain and evolve tests as features change over time.
Frameworks like PHPUnit are constantly updated to align with modern practices. Most frameworks adopt a model-driven or risk-based testing approach. These methodologies prioritize risks related to user satisfaction versus the technical risks involved.
A notable venture into this arena is the continuous feedback improvements. Integration of tools such asLaravel Shift allow automated code upgrades and building tests alongside CRM changes. Furthermore layouts for documentation, such as ASCII values to frame test cases, play crucial role in helping teams adopt standards needed in their workflows.
In summary, by being aware of these emerging trends and evolving standards, developers can better prepare for the future landscape of PHP unit testing. This, in turn, can enhance team productivity, cross-functional communication, and ultimately lead to higher quality software.
As PHP development continues to advance, so too must our approaches to unit testing remain relevant, focused, and continuously optimized for enhanced delivery.