Mastering Python Classes and Objects for Effective Programming
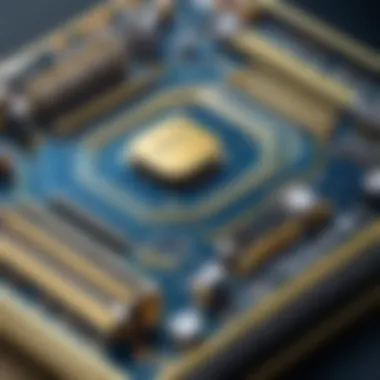
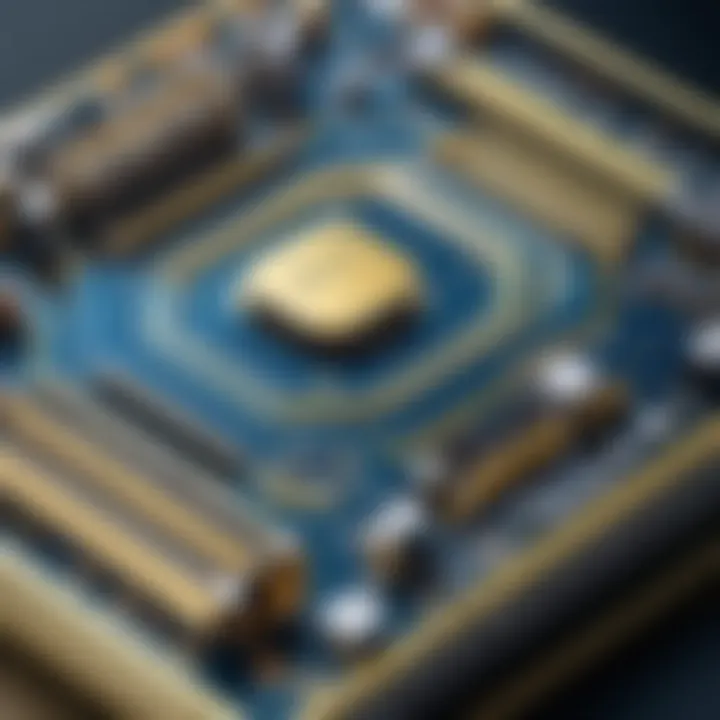
Intro
In the landscape of programming, understanding classes and objects is fundamental, especially when utilizing Python. This article aims to clarify these concepts while linking them to broader themes like encapsulation and inheritance. Through practical examples, both aspiring and experienced programmers will develop a solid grasp of object-oriented programming principles.
Python's approach to classes and objects is succinct yet surprisingly powerful. The essence of these constructs is crucial, not only for writing clean code but also for fostering reusable software components. In this exploration, we will dissect various facets that enable the programmer to leverage Python's full potential.
Important Note: Mastering classes and objects opens the door to cleaner, more maintainable code, ensuring your programs are both efficient and scalable.
Now, let’s delve deeper into specific topics, ensuring an enriching experience for the targeted programming enthusiasts.
Coding Challenges
Coding challenges are an excellent way to solidify the understanding of Python classes and objects. Engaging with complex problems fosters critical thinking and a profound comprehension of how object-oriented programming elevates coding practice.
Weekly Coding Challenges
Each week, programmers should tackle unique coding challenges that necessitate the use of classes. Problems could range from simple design of a class managing contacts to more intricate designs like a multiuser messaging application built using classes.
Problem Solutions and Explanations
It's important to analyze solutions to past challenges to comprehend how others utilize classes efficiently. Understanding various solutions provides insight into different programming styles and emphasizes the significance of creativity in finding solutions.
Tips and Strategies for Coding Challenges
- Break Down Problems: Before jumping into code, analyze the challenge thoroughly and break it into smaller parts.
- Consider Object Interactions: Outline how different classes will interact with each other.
- Implement Incrementally: Start small by implementing essential methods before the complete class structure.
Community Participation Highlights
Many platforms host avid programmers exchanging insights. Engaging with the community offers diverse viewpoints and methods related to specific coding challenges. Observing others can refine your own coding approach, enhancing your skills exponentially.
Preamble to Object-Oriented Programming
Object-Oriented Programming (OOP) is an essential paradigm within software development. It introduces a distinct approach to programming by focusing on the interaction and collaboration between various objects. This methodology can significantly enhance code organization, scalability, and reusability. As programmers develop complex applications, understanding OOP becomes crucial. Python, among many programming languages, embraces OOP principles beautifully.
The appeal of Python's object-oriented capabilities is grounded in its core characteristics. By facilitating the modeling of real-world scenarios through classes and objects, developers can create more intuitive and manageable code. This article begins by leading readers through a structured definition of OOP, then discusses its key principles, providing a foundational understanding essential for any developer.
Definition of Object-Oriented Programming
Object-Oriented Programming is a programming paradigm that uses
What are Classes in Python?
Understanding classes in Python is essential for grasping object-oriented programming. Classes serve as blueprints for creating objects. Each class can define attributes and behaviors that the objects derived from it will carry. This concept provides a wildlife of organization and reusability in coding. It allows programmers to construct distinct data structures, each modeled after real-world entities, complete with properties and functions.
Definition and Purpose
In the realm of Python, a class is defined as a user-defined blueprint or prototype from which objects are created. Classes encapsulate data for the object and define functions that manipulate this data. The primary purpose of a class is to bundle data and functionality together, easing programming complexity.
Creating a class allows for a systematic way to construct objects, making it easier for developers to maintain and expand their code. Python classes lay the groundwork that allows for properties—commonly known as attributes—as well as methods, which are essentially functions associated with the class.
The Structure of a Class
When working with classes in Python, understanding their structure is key. A well-structured class enhances clarity and usability in programming. The main components of a class include class attributes, instance attributes, and methods.
Class Attributes
Class attributes are variables that are shared across all instances of a class. They are declared directly within the class but outside any methods. A fundamental characteristic of class attributes is that when modified, they reflect the change across all object instances unless overridden by instance-specific attributes. This makes class attributes popular for defining constant values shared among instances.
One significant advantage of class attributes is their ease of use. Developers can define behaviors or characteristics that apply to all objects at once, promoting efficient memory consumption. However, a key disadvantage lies within shadows properties ranges, where instance variables might be preferred if unique behavior is required per object.
Instance Attributes
Unlike class attributes, instance attributes are specific to each object created from the class. They allow each instance to maintain its own state. An instance attribute is assigned usually within a class's method, often within the constructor. The uniqueness of each attribute lies in its ability to preserve distinct values for different objects.
Instance attributes are beneficial for representing dynamic properties or states of each object. They create a personalized experience for every object instance, enabling customized interactions. On the flip side, they rely on additional memory for each instance, which must be planned for when designing classes with many permutations.
Methods
Methods can be thought of as functions that are defined inside a class. These functions govern the actions that can be performed on objects of that class. A vital characteristic of methods is that they have at least one parameter, typically identified as , allowing self-reference to the object on which the method is called.
The presence of methods is crucial for encapsulating behaviors, thus forging a stronger interaction model between attributes and operations. They facilitate code reusability, as the same method can be called on different instances with distinct data. However, careless use of methods can introduce complexity, leading to deeper and problematic class hierarchies.
To summarize, understanding how to structure a class effectively, with its attributes and methods, is an invaluable skill for proficient programming in Python. The construction of well-formed classes plays a significant role in ensuring code maintainability and clarity.
Defining a Class in Python
Defining a class in Python is a critical aspect of object-oriented programming. It brings structure and modularity to the code. A class can be thought of as a blueprint for creating objects. Each object created from the class will inherit properties and methods defined in the class. Thus, defining a class is not just about writing some code; it is about creating a pathway for an efficient work framework.
When you define a class, you are able to encapsulate data and functionality together. This is important because it allows for a much clearer organization of code as compared to using functions alone. By grouping related attributes (data) and methods (functions) together, it becomes easier to manage complexity.
Key benefits of defining classes in Python include:
- Reusability: Once a class is defined, it can be reused throughout your code without rewriting the same logic.
- Maintainability: Changes to functionality can often be made in one location, the class, rather than tracking down many instances across the codebase.
- Abstraction: Classes provide a way to hide complex logic and data, exposing only what is necessary to the user.
- Flexibility: As your code grows, you can create new classes that inherit from existing ones, allowing for more dynamic and understandable code bases.
It is essential to consider naming conventions when defining classes. In Python, classes are usually named using , which makes them easily distinguishable from functions and variables.
Syntax Overview
Defining a class in Python follows a straightforward syntax. A typical class definition starts with the keyword, followed by the class name and a colon. This structure organizes the content effectively and makes it comprehensible.
Example of basic syntax:
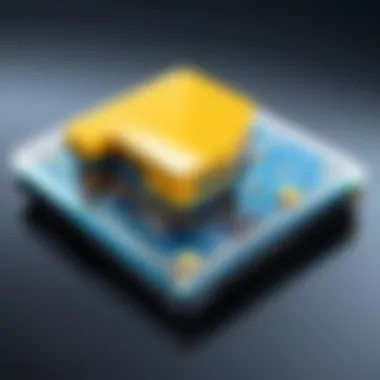
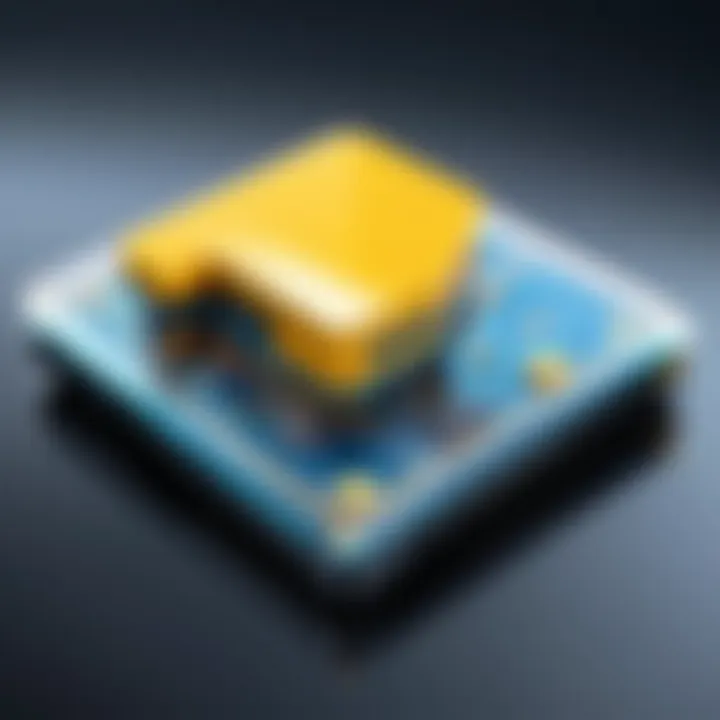
In this structure:
- The keyword begins the definition.
- designates the name of your class, which should be descriptive of its function.
- is the constructor method called when an object of the class is created. It is used to initialize instance variables.
- Methods can contain various functions to operate on the attributes within the class.
Creating a Simple Class Example
Creating a simple class serves as a practical introduction to working with Python classes. Here’s an illustration of a basic class:
In this simple example:
- is the class name.
- The constructor method initializes three attributes: , , and .
- is a method that returns a string representation of the car.
To utilize this class, one would instantiate an object as follows:
This implementation covers the essentials of defining a class leaving space for expansion and more complex functionalities. As coding practices go, understanding how to define a class is foundational for advancing to more complex principles in Python.
Creating Objects from Classes
Creating objects from classes is fundamental in programming with Python. It allows developers to leverage the distinct attributes and behaviors defined within a class. Understanding how this process works can greatly enhance your software development skills and improve code organization and reusability.
Instantiating Objects
Instantiating objects refers to the creation of a specific instance of a class. An object serves as the tangible representation of the class itself, comprising attributes and methods encapsulated in that class. The importance of instantiation lies in ensuring that every object can maintain its own set of values, which are held in its attributes. This modular approach enables programmers to develop complex applications in a more organized manner. Numerous instances of the same class can coexist, each with distinct state and behavior, thereby enabling various functionalities without mixing input or output.
Constructor Methods
Constructor methods are special methods invoked when creating a new object. Its primary purpose is to initialize attribute values when the object is instantiated. The standard Python approach uses the method as a constructor.
Method
The method plays a vital role in defining how an object should be set up just after it has been created. This constructor allows hardware to assign values to instance variables. One key characteristic of the method is its method signature, which always includes , the reference to the current object being created. This makes it possible to differentiate instance attributes from local variable in, for example, calculations.
The unique feature of the method is that it can accept additional parameters. This flexibility allows the user to provide initial values as arguments upon instantiation. Therefore, developers can choose default values, reduce the need for later modifications, or ensure consistent objects in behavior as needed. Regardless, potential drawbacks compдинg with this flexibility can emerge if default values lead to bugs or if users pass values that maintain incompatible states. Thus, using wisely is crucial for maintaining any overall application stability.
Parameters and Arguments
Parameters and arguments play an essential part in creating initialized objects. In the context of the method, a parameter refers to the variables defined within the method, which receive values when an instance is created. Arguments, on the other hand, are the real values passed to the method during object instantiation.
Employing parameters and arguments immensely betters code readability and maintainability. It is a beneficial choice because programmers can create objects with varying properties upon creation simplifying design patterns. A common use-case for parameters is controlling access to certain functionalities to pre-define allowed values, thus preventing misuse.
The unique feature regarding parameters and arguments is the scope they restrict; private member variables get their values at the construction phase. However, misuse of balance during longer parameter lists might contribute to complexities or higher chances of error during instantiation. Therefore, maintaining manageable parameter lists is a best practice.
:memo: Using constructor methods effectively increases the value of the classes you develop by providing necessary initial conditions.
To conclude, understanding the mechanisms behind object instantiation lays the groundwork for effective object-oriented programming in Python. When you learn these concepts, embrace each structured element through exploratory implementation. Practicing instantiation broadly increases application complexity with control and accuracy.
Accessing Attributes and Methods
Accessing attributes and methods is a critical component of working with classes and objects in Python. This section emphasizes its significance in understanding the behavior of objects within object-oriented programming. By mastering the method of accessing these components, programmers can manipulate and utilize data effectively, creating dynamic programs that adapt to various functional needs. This topic also lays the groundwork for principles that enhance code reusability and efficiency, essential in complex applications.
Dot Notation
Dot notation serves as the primary means of accessing attributes and methods in Python. It involves the use of a dot () followed by the attribute or method name after the object reference. This straightforward method brings clarity. For instance, consider a class named :
Here, accesses the attribute from the object instantiated from the class.
Using dot notation also applies to methods defined within the class. For example:
When you call , it triggers the method associated with the object.
This clear syntax allows for easy readability and modification of attributes and methods. Thus, understanding dot notation is fundamental for programmers who want to navigate Python's object-oriented features effectively.
Modifying Attributes
Modifying object attributes is pivotal for customization and dynamic behavior. Through dot notation, attributes can be easily altered after creating instances of the classes. This flexibility increases the program's adaptability without needing to reinitialize objects.
Consider the following code:
In this example, the attribute of changes from
Understanding Class and Instance Variables
In this section, we will discuss the distinction between class variables and instance variables, two crucial concepts in Python that influence how data is stored and accessed within classes and objects. Understanding these differences ensures efficient design and manipulation of data related to object-oriented programming, which significantly affects how developers manage their coding practices.
Difference Between Class and Instance Variables
Class variables and instance variables serve different purposes within a class. Class variables are defined within the class itself and are shared among all instances of the class. If the value of a class variable is changed in one instance, it impacts all instances of that class, reflecting a collective state. This characteristic is essential when the variable represents a property that should maintain a singular state across many objects, such as a constant.
In contrast, instance variables, which are defined within the constructor, are unique to each object instance. When an instance variable is assigned, it only affects that particular object, allowing different objects to hold different data. This feature is particularly beneficial when ensuring that each object maintains its unique attributes rather than sharing a generic one.
Comparison of Class and Instance Variables
- Ownership: Class variables belong to the class itself, while instance variables belong to individual objects.
- Shared State: Class variables are shared among all instances, but instance variables can differ in every instance.
- Declaration: Class variables are declared within the class but outside any methods, while instance variables are typically initialized inside the method (constructor).
Practical Examples
To solidify the understanding of class and instance variables, we can look at practical implementations. Here’s a simple code snippet illustrating both types of variables.
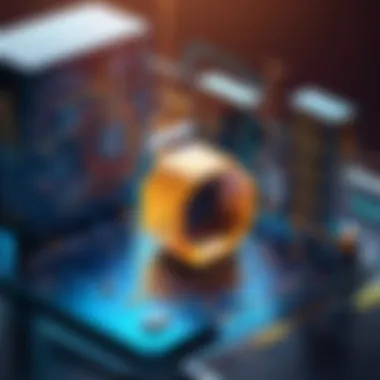
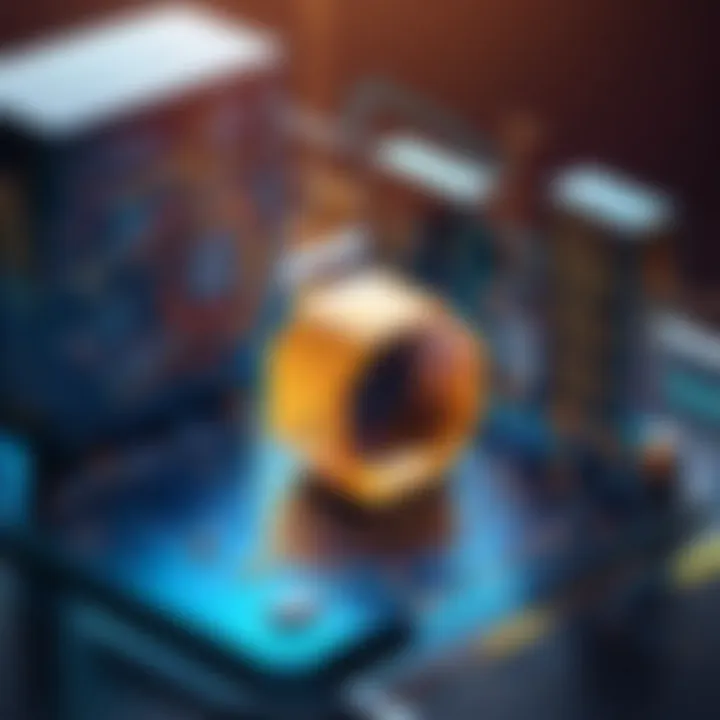
In the code above, is a class variable that tracks the total number of instances created. Every time a new object is instantiated, this variable is updated. On the other hand, and are instance variables, ensuring that each car can have distinct characteristics.
Using both class and instance variables effectively allows developers a high level of control and organization in their classes. Proper use of these variables will enhance code maintenance and functionality.
Inheritance in Python
Inheritance is a core concept in Object-Oriented Programming (OOP), especially in Python. It allows one class to inherit the attributes and methods of another class. This facilitates code reuse and enhances organization in your programs. Inheritance also creates a natural hierarchy within the object structure. It lets programmers create a new class based on an existing class, reducing redundancy.
What is Inheritance?
Inheritance is like a family tree for classes. A child class inherits the properties of a parent class. This relationship allows the child class to use, modify, or extend the behavior defined in the parent class. If a developer modifies the parent class, the child class automatically reflects those changes. This inheritance chain forms a powerful basis for building functionality without unnecessary duplication.
Types of Inheritance
Inheritance can take several forms, each with unique characteristics and implications. Understanding these can significantly enhance a programmer’s use of the OOP paradigm in Python.
Single Inheritance
In single inheritance, a subclass derives from one and only one superclass. This structure simplifies the hierarchy and enhances clarity. The key characteristic is simplicity; it allows for easy understanding of relationships between classes. By following this model, you avoid complications that arise in more complex systems. A primary benefit is reduced potential for errors related to method resolution.
Advantages: Simplicity in design, straightforward relationships, and ease of debugging.
Disadvantages: Limited flexibility since the subclass can only extend one superclass.
Multiple Inheritance
Multiple inheritance allows a child class to inherit from more than one parent class. This provides increased flexibility and expressiveness. A notable characteristic is the ability for a subclass to implement behaviors from multiple sources, thus emulating real-world scenarios more accurately. However, it can become complex, mainly when methods from different classes conflict, resulting in ambiguity.
Advantages: Increased possibilities for functionality, and modeling interrelated concepts more effectively.
Disadvantages: Higher complexity and potential for conflicts in methods from various parents.
Multilevel Inheritance
In multilevel inheritance, a class can inherit from another class, which in turn inherits from another class. This can create a more tiered class structure and allows for a detailed organization of entities. The uniqueness lies in creating chains of inheritance where benefits and attributes are passed through multiple levels. This structure enhances versatility in designs.
Advantages: Improved organization by breaking down complex systems, and allows gradual specialization.
Disadvantages: If overused may lead to complicated inheritance chains that challenge readability and understanding.
Utilizing inheritance in Python, therefore, not only streamlines your process of developing classes but also offers potent programming patterns that foster easier maintenance and scalability of code, critical for any thriving project.
Encapsulation in Python Classes
Encapsulation is a core principle in object-oriented programming that plays a pivotal role in Python classes. It refers to the bundling of data and methods that operate on that data within a single unit or class. This approach restricts direct access to some components, which can prevent the accidental modification of internal data and enhance the security of the program. Encapsulation contributes to cleaner, more maintainable code, as well as easier debugging.
One vital aspect of encapsulation is how it helps in managing the visibility of data. By exposing only the necessary components through defined interfaces, encapsulation enables enforcing invariants and controlling behaviors of objects effectively. Furthermore, encapsulation promotes separation of concerns, where specific functionalities and data queries are identified and managed independently. This results in reduced code complexity, making it easier to understand the interactions within an application.
Purpose of Encapsulation
The purpose of encapsulation extends beyond merely packaging data and methods. It is fundamentally about managing complexity and safeguarding sensitive data. By simplifying the interactions between different parts of a program, encapsulation leads to higher code quality and minimizes the dependencies, which is crucial in larger programs.
Additionally, encapsulation allows for controlled access to class attributes. This is particularly useful when there is a need to validate data before assignment or when modifications require additional actions. Simplifying user interface while retaining functionality is often a key goal of encapsulation.
Access Modifiers
In Python, the visibility of attributes is mainly managed through access modifiers. These modifiers serve to inform users of a class about how they are expected to interact with its properties and methods. There are three primary types of access modifiers: public, protected, and private. Each plays a unique role in determining the accessibility of class members.
Public
The public access modifier allows unrestricted access to class attributes and methods. This means any part of a program can use public members directly without any restrictions. It's a popular choice because it simplifies usage; programmers can interact with the features of a class freely.
Key characteristics of public members include:
- Accessibility: Public attributes and methods are accessible from outside of the class.
- Affordability: Easy to implement, examine, and interact with, aiding rapid prototypes.
While public access can facilitate easier development and debugging, care must be taken to ensure that essential parts of the system do not become exposed unnecessarily, leading to unintentional changes.
Protected
Protected access modifiers provide slightly more control compared to public members. It indicates that a class member is intended to be accessible only within its own class and subclasses. This creates a layer of encapsulation; the member cannot be accessed outside this hierarchy.
Key characteristics of protected members include:
- Limited Accessibility: Not meant for outside access, the protection protects the integrity of the data.
- Subclass Access: Allows derived classes to utilize protected members when inheriting.
While using protected members can help enforce certain constraints, over-reliance on them can complicate class design, particularly in scenarios with multiple levels of inheritance.
Private
Private members serve to completely restrict access from outside of the class. By prefixing an attribute with a double underscore, Python achieves a form of strong encapsulation. This makes it easy to hide internal structures from the outside world while providing controlled access via public or protected methods.
Key characteristics of private members include:
- Strict Control: Ensures no direct external modifications, enhancing data integrity.
- Encapsulation: Enables class designers to hide complexity by maintaining internal logic away from external interaction.
However, using too many private members can make interaction with the class cumbersome, often requiring explicit access methods, complicating usage unnecessarily. It's crucial to strike a balance between necessary encapsulation and usability.
Conclusion: In encapsulating class members using public, protected, and private modifiers, programmers enhance data security and code maintainability, vital aspects when developing robust Python applications.
Polymorphism in Python
Polymorphism is an important concept in object-oriented programming, and it plays a critical role in Python classes and objects. This feature allows methods to perform similar functions in different contexts. Understanding polymorphism can enhance code reusability and flexibility. In practice, it enables subclasses to process requests from methods overriding or interfaces in a unified way, making systems more coherent and versatile.
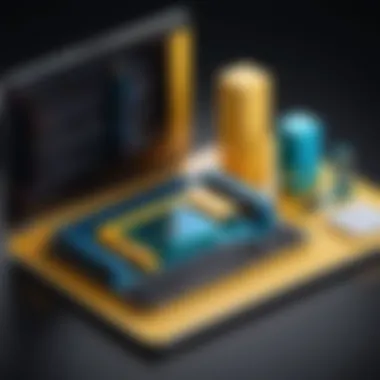
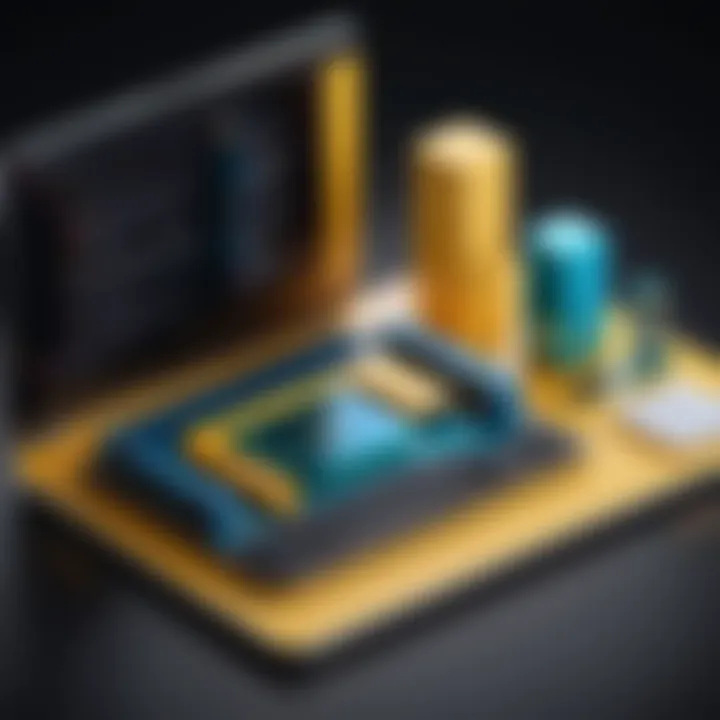
Understanding Polymorphism
In its simplest form, polymorphism means "many shapes". It's a principle that enables objects of different classes to be treated as objects of a common superclass. Due to polymorphism, a single interface can represent different behaviors based on the objects that implement it. This leads to easier code management and allows programmers to write more generic and reusable code snippets.
Polymorphism primarily manifests in two forms: method overriding and method overloading. In Python, however, we focus more on method overriding. With Python's dynamic typing feature, it allows functions and classes, regardless of their types, to be utilized interchangeably.
Method Overriding
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This functionality allows the derived class to offer more tailored behavior compared to its parent class.
To illustrate, consider the following simple example:
Output:
In this example, both and have a method that overrides the method from . When we iterate through the list of and call , the proper method is called based on the object's actual class. Thus, polymorphism provides a clear structure which aids in code extension and adaptability, allowing programmers to modify subclass implementations without affecting the parent class.
This fundamental capability of polymorphism enhances scalability in applications and allows programmers to build upon existing code with minimal disruptions to system behavior, emphasizing its significance in modern programming practices.
Abstract Classes and Interfaces
Abstract classes and interfaces play a pivotal role in the hierarchy of Python programming and object-oriented design. They provide foundational structures that promote code reuse and maintainability, which are critical in complex systems. When designed thoughtfully, both abstract classes and interfaces can simplify interaction between components in a software project, making interactions explicit and manageable. In addition, these concepts encourage more organized programming through established contracts for object behavior.
Although they serve similar purposes, abstract classes and interfaces have distinct characteristics that can either enhance or limit their use depending on the scenario. Both guide developers in how classes should function and what methods must be implemented. Nevertheless, it's essential to grasp their differences and applications fully to utilize them effectively in projects.
"Abstract classes and interfaces spark coherent design in programming by establishing expectations for derived classes."
Role of Abstract Classes
Abstract classes serve as blueprints for other classes. They cannot be instantiated directly and are primarily intended to define methods that must be created within any child classes. Due to this abstract nature, the designer can enforce certain standards without providing fully functional implementations. For example, let's consider a hypothetical game development scenario with a base class called . This class could define abstract methods such as and , which every derived character type must implement.
Benefits of using abstract classes include:
- Code Resusability: Highly promotes the reuse of code, as shared attributes and methods can reside in the abstract class.
- Standardization: Ensures that all derived classes provide necessary methods, thereby establishing a common interface for a group of related classes.
- Flexible Design: Developers can create multiple child classes that might offer different implementations of the same method, thereby enhancing design patterns.
By guiding child classes, abstract classes maintain a coherent structure that retains flexibility in functionality.
Creating an Interface in Python
Creating an interface in Python can be achieved through abstract classes with the help of the module, for abstract base classes. Typically, an interface will not provide any concrete methods; it will only declare them without implementation. This ensures that any class expecting to implement the interface must provide all the methods it describes.
Here's a simple example:
In this code, is an abstract class with abstract methods and . The class implements those methods. This setup ensures any class derived from will adhere to a specific suit of behaviors, which aids in designing large systems where multiple components interact.
Common Pitfalls in Using Classes and Objects
Understanding the common pitfalls in using classes and objects is essential for effective Python programming. Misunderstandings in these areas can lead to inefficient code, unexpected behaviors, and project delays. Awareness of these pitfalls enhances not only code quality, but also development speed. By addressing these issues early on, programmers can avoid frustrations and pitfalls later.
Misunderstanding Scope
Scope refers to the visibility and life cycle of variables and methods within a Python program. A common mistake made by programmers is the confusion surrounding the scope of instance variables and class variables. Instance variables, assigned within methods, belong to a specific instance of a class. In contrast, class variables are shared across all instances of that class.
For example, when creating methods, programmers must understand that using refers to an instance variable, while simply using without will reference a variable in the current scope. If the variable exists outside of the class, it will not be accessible unless properly scoped. This can lead to surprising behavior especially when a programmer expects to modify a class variable but unintentionally alters an instance variable instead.
Mentioning scope concerns may underline the importance of documentation within your code. Clearly explaining what each variable represents, especially in complex class structures, aids others—and your future self—in understanding the functionality behind each segment.
Overusing Class Hierarchies
Python encourages the use of inheritance, allowing classes to inherit properties and methods from base classes. However, overusing this feature may lead to deeply nested inheritance structures that complicate code unnecessarily. This style can hinder readability and maintainability. Developers often forget that simpler is better—especially in coding.
Following the principles of sound object-oriented design is necessary to use inheritence effectively. Be cautious of class hierarchies beyond two or three levels as thier usage usualy requires extra attention with respect to references. In practice, it's always wise to consider composition over inheritance. This means applying objects within others’ instances rather than creating overly complex hierarchies.
Encouraging a clear class laayout comes together when defining ther functionalities central to project requirements. Strive for clarity by ensuring that each class serves a distinct purpose. When in doubt, reconsider if a new class is needed or if an existing class can accommodate further functions.
Composition allows distinct functionality while maintaining independence, achieving better encapsulation and flexibility.
By avoiding these common mistakes associated with scope and excessive class hierarchies, programmers enhance both project clarity and speed of development. Awareness and constant evaluation of one's code make a noticeable impact on overall project architecture.
Culmination
The conclusion of this article serves to summarize the foundational elements discussed throughout the exploration of Python classes and objects. It plays a crucial role in reinforcing reader understanding of object-oriented programming principles. The significance lies in clearly communicating the overall benefits and applications of what has been learned.
By reiterating key concepts such as encapsulation, inheritance, polymorphism, and the structure of classes, this section helps solidify the reader's grasp of OOP. These topics provide practical techniques that inform effective programming. Thus, the conclusion is not merely a restatement, but an essential tool for elevating learning outcomes.
In practical terms, embracing these principles enhances code quality and maintainability. Concise classes make it easier to modify and extend functionality over time, which is beneficial as projects scale. OOP reduces redundancy, allowing programmers to focus on unique aspects of class design.
Recap of Key Concepts
This section of the conclusion encapsulates the central ideas dissected in the in-depth examination of classes and objects. To ensure clarity, key points include:
- Concept of Classes and Objects: A class is a blueprint for creating objects. Objects are instances of classes, encapsulating data and behavior.
- Attributes and Methods: Classes have attributes that define properties and methods defining actions. Combining these allows for modeling real-world entities.
- Importance of Encapsulation: Encapsulation promotes restricting direct access to some class attributes. It safeguards object integrity by limiting exposure to the internal state.
- Inheritance and Polymorphism: Inheritance fosters code reusability, and polymorphism allows for method overriding, making the codebase flexible and easier to maintain.
These pillars of object-oriented programming establish a pathway for programmers to implement better, cleaner code.
Future Directions in Python Programming
The landscape of Python programming continues to evolve, influencing how classes and objects are used in upcoming projects. A few future trends should be taken into consideration for staying competitive:
- Increasing Emphasis on Type Hints: As Python's popularity grows, the community increasingly adopts type hints, enhancing code clarity and allowing for proper error checks.
- Growing Relevance of Data Classes: Python's data classes simplify class definitions with automatic features like comparison and representation customizations. These tools encourage succinct coding practices.
- Integration with Modern Frameworks: Frameworks such as FastAPI and Django leverage class-based views, promoting a more structured approach around classes, signaling a continual shift towards neighboring disciplines.
With continuous advancements in the Python ecosystem, programmers are recommended to stay informed about these developments ensuring their skills remain relevant.
Overall, the conclusion serves as more than an endpoint; it highlights critical thinking around built knowledge while propelling programmers toward future opportunities in Python development.