Mastering Python Dataclasses: A Complete Guide
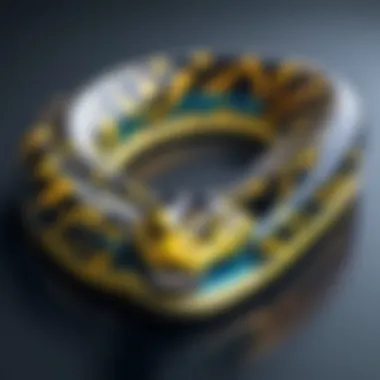
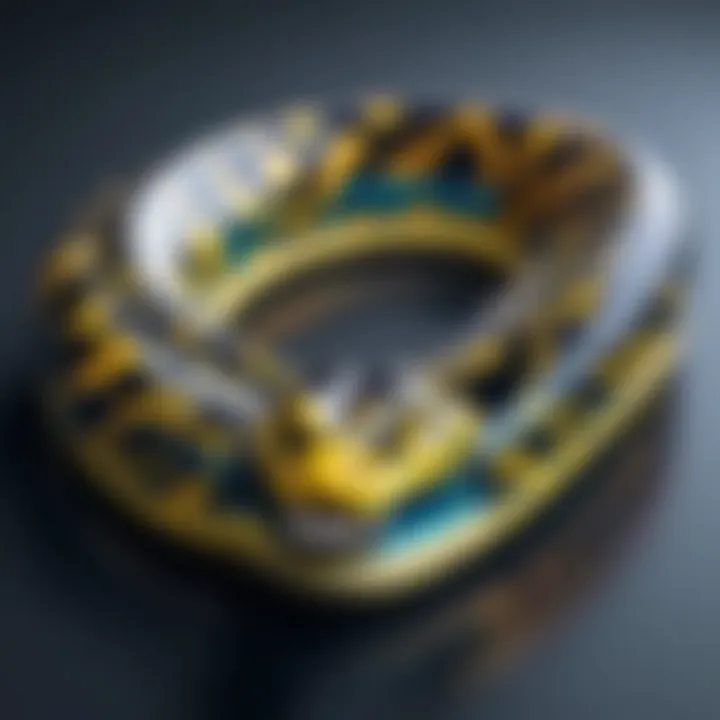
Intro
Python, as a programming language, is well-known for its simplicity and effectiveness. The introduction of dataclasses in Python 3.7 is a testament to this philosophy. Dataclasses provide an elegant and efficient approach to create classes primarily meant for storing data. This is particularly useful when developing applications that need clear structure and organization of data.
Dataclasses reduce boilerplate code associated with creating classes by automatically generating important methods such as , , and . This not only saves time but also enhances code readability, making it easier for other developers to understand your intentions easily. Importantly, using dataclasses can improve efficiency in coding practices as a result.
As we dig deeper, the article will present various aspects of dataclasses in Python. We will break down their syntax and examine practical applications in programming. In doing so, we'll highlight their performance implications and integration with other coding techniques. Overall, the intent is to present a clear picture of when and how to leverage dataclasses in computer programs effectively.
Prelims to Python Dataclasses
Python dataclasses offer a noteworthy advancement in how data is handled within Python programming. They streamline the creation of classes specifically tailored for data storage, substantially relaxing the complexity involved in managing data-centric code. The design of dataclasses introduces inherent advantages, such as automatic generation of methods that aid in comparison, representation, and data manipulation, all with minimal code clutter.
These benefits shape not only code readability but also the maintainability of software projects. In essence, the standard programming process boasts an ineffective, verbose approach when users engage with traditional classes. This presents a compelling case for the incorporation of dataclasses into regular practices.
Considerations surrounding the use of dataclasses extend beyond mere syntax changes. By understanding their definitions, applications, and use cases, developers can leverage Python’s enhanced capabilities, making their programming endeavors more efficient. Whether creating basic applications or high-performance systems, recognition of dataclasses' importance is paramount.
Definition and Purpose
Dataclasses in Python serve as boilerplate reduction tools. Introduced officially in Python 3.7, a dataclass automatically generates special methods based on class attributes. Consequently, decision-making regarding data structures becomes more straightforward without writing incessantly repetitive code.
The primary purpose of dataclasses is to allow the straightforward creation of less error-prone classes that mainly hold data. At its core, the objective is to enable easier management and handling by reducing unnecessary complexities and enhancing focus on individual aspects of data structures.
For instance, if designing a class that represents a point in two-dimensional space, defining it via a conventional class would involve clearly stating its attributes, writing initializers, grudgingly creating equal and string methods, and potentially more. A dataclass permits all of the prior pretensions through some deft appraisal of attributes alongside specified types—clean and accommodating.
Example:
Here, one again sees how defining a class translates minimalistically with the dataclass approach.
History of Dataclasses in Python
The inception of dataclasses is rooted in the growing need for straightforward class definitions and data manipulation in programming practices. Before Python 3.7, programmers faced challenge often unquestioningly accepting cumbersome tasks. While property decorators and init, methods existed to aid in initializing class attributes, the repetitive nature of coding continued to dominate.
With an inherent desire for efficiency, a new vision emerged within the Python community. Discussions within various forums led to drafts that culminated in PEP 557, which set the course for integrating this much-desired functionality into the subsequent Python versions.
Significant focus during the evolution of this feature involved pinpointing usability, reducing code clutter while enriching functional attributes. The initiative eventually received sufficient momentum and official recognition, propelling dataclasses into the foreground as new standard tools in class designing post-2020 frameworks—to define code succinctly.
The severe practicality provided by dataclasses has established them not only as favored choices for developers but also as practical replacements to lesser flexible constructs. Knowing this history reveals that the journey of datatype representation within Python carries substantial notion, bridging gaps that existed in coding practices since altogether evolved-state started settling in the realm of structured hierarchies.
Syntax of Dataclasses
Understanding the syntax of dataclasses is essential for any programmer looking to effectively utilize this feature in Python. The syntax allows developers to define data-centric classes swiftly. Specific elements within this syntax streamline the development process and enhance code clarity, making the codebase more maintainable.
Basic Structure
A dataclass is defined using the decorator, placed above a class definition. The simplest form would look like this:
In this example, the class automatically receives an initializer that takes and as parameters. This concise syntax emphasizes simplicity in creating classes primarily for storing data without boilerplate code.
This straightforward approach is significant for maintaining focus during development. Avoiding verbose class definitions makes it easier for programmers new to dataclasses to grasp the structure quickly and reduces the chances of errors.
Automatic Generation of Special Methods
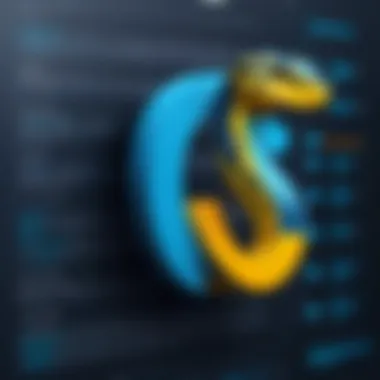
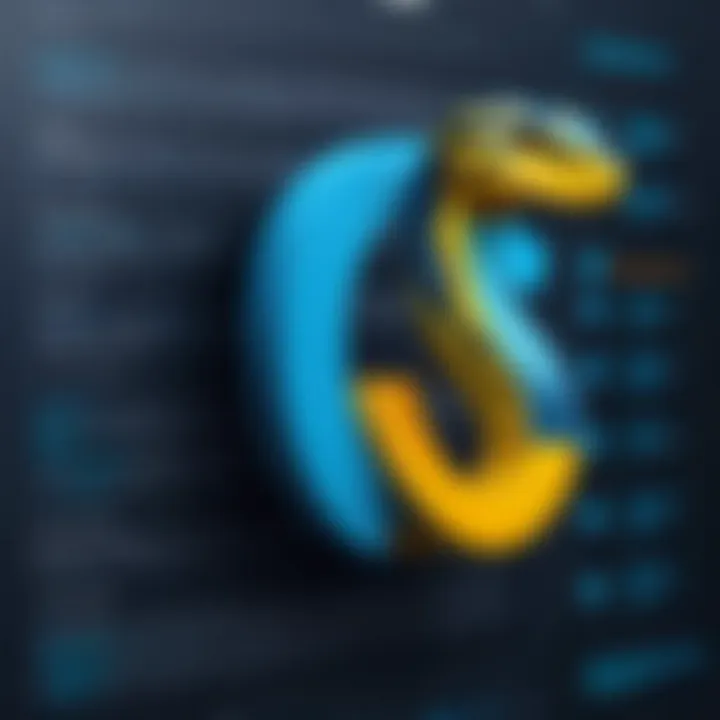
One of the remarkable benefits of using dataclasses is the automatic generation of special methods. When Python encounters a class decorated with , it generates several methods. These include , , , and others, based on the defined fields of the class.
As a developer, this means a few interesting things:
- Reduced Boilerplate: There is no need to create the initialization method manually, allowing you to devote time to other aspects of project development.
- Built-In Safety: Comparing instances is handled transparently, thus reducing manual work while also decreasing potential human errors that might arise from implementing these methods by hand.
- Ease of Maintenance: Updated field names are automatically adjusted in these generated methods, making refactoring smooth.
Staying aware of this automatic generation during the development process ensures optimal use of dataclasses.
Field Specification
Field specification gives users more control when working with dataclasses. It is achieved through using the function, which offers options on various aspects of a field's behavior.
This can be quite beneficial when you want to add default values or when dealing with factory functions for complex data types. Here’s a closer look:
In this particular example, takes a random value between 0 and 100 if not otherwise provided. The syntax acts as a gatekeeper, helping define rules and constraints while maintaining the integrity of data Structures.
Furthermore, field specification opens doors for more advanced tasks such as type validation and enforcing constraints. Understanding this capability can greatly improve the robustness of code architecture when implementing data classes.
The syntax of Python dataclasses combines simplicity with powerful features, allowing developers to swiftly manage data storage and integrity.
Key Features of Dataclasses
Understanding the key features of dataclasses is essential to appreciate their role in Python programming. These features streamline code reduction while enhancing readability. This section presents significant aspects of dataclasses, focusing on immutability support, default values, factories, and comparison methods. Each element showcases how developers can leverage dataclasses to create cleaner, more maintainable code.
Immutability Support
Immutability provides distinct advantages in programming. With dataclasses, declaring a class as immutable allows for easier reasoning about state transitions. An immutable dataclass can be created by setting the 'frozen' parameter to . This way, instances of that dataclass cannot be altered after initialization, making them particularly useful for scenarios such as concurrent programming where thread safety is critical.
With immutability, one can safely pass around data structure instances without worrying about unintended side effects. While immutability might add some overhead in handling updates, the trade-off often leads to higher consistency and fewer bugs.
Default Values and Factories
Dataclasses allow developers to specify default values for class attributes which enhance flexibility at the time of instantiation. Defined default values streamline initialization by removing unnecessary arguments in constructor calls. In more complex scenarios, utilizing default factory functions allows for dynamic default values that can vary per instance, further tailoring the instantiation process.
In the example above, if no age is provided, it defaults to 30. The use of for lists ensures that each instance has its unique list. Owning such flexibility enriches class functionality.
Comparison Methods
Another notable feature is the automatic generation of comparison methods. By simply using the decorator, Python generates methods like (equality), (less than), and others. This automation significantly reduces repetitive code and minimizes potential errors in manual definition of these methods.
By employing generated comparison methods, psychologists or configurable structures become easier to work with. Developers can focus on the core functionalities of class logic rather than boilerplate code.
Overall, these key features of dataclasses not only save time but also encourage developers towards a cleaner and more maintainable codebase. When implemented properly, they transform traditional class structures into more flexible and effective solutions.
Practical Applications of Dataclasses
The practical applications of dataclasses are extensive and significant. They enhance data handling, simplify code structure, and bring clarity to what could otherwise be a complex implementation. As programmers grasp the utility of dataclasses, they will discover various scenarios where this feature can bring tangible benefits. In this section, we will explore important practical uses for dataclasses, specifically in data validation and managing configuration settings for projects.
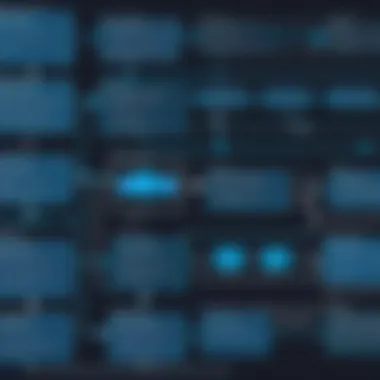
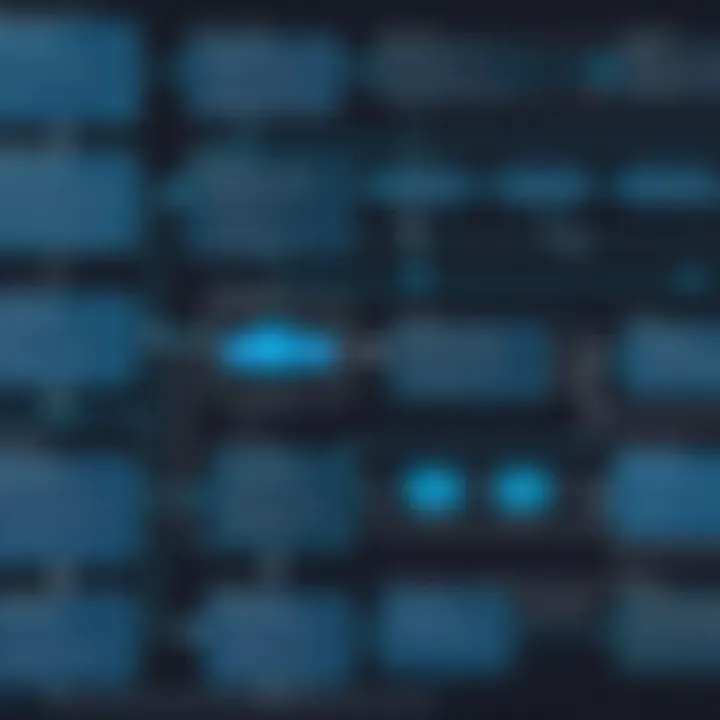
Data Validation in Web Applications
Data validation is critical in web applications. Ensuring that the input from users meets certain standards prevents potential security issues and helps maintain data integrity. Dataclasses have become an advantageous tool in this context due to their structured and clear nature.
Using dataclasses, developers can define expected types and constraints associated with user inputs. For example, if a certain input needs to be an integer within a defined range, a dataclass can be employed to encapsulate that input requirement swiftly. Apart from enhancing code aesthetics, implementing dataclasses in this manner promotes better organization of validation logic.
Example of Validation with Dataclasses
In this example, a dataclass is defined with fields for , , , and . The method checks basic validation criteria for age and email. With this model in place, validating user inputs becomes more organized and easier to manage.
Configuration Classes for Projects
Managing configurations often proves troublesome, with developers struggling to maintain clarity and structure. Dataclasses offer a straightforward and clean approach to handling configurations. They simplify interaction with configuration data and help ensure all necessary fields are defined.
As projects grow in complexity, representing configuration settings via dataclasses allows for a centralized and systematic way of managing project settings. This creates a more readable, concise structure that minimizes potential for error.
Benefits of Using Dataclasses for Configuration
- Type Safety: Each configuration option is clearly defined, improving safety by preventing incorrect data types.
- Defaults Handling: Default values for configurations can be defined easily, minimizing boilerplate code.
- Easy to maintain: As the configuration needs of a project expand, dataclasses can be updated or extended logically.
Example of a Configuration Class
This simple approach presents an elegant solution for managing configuration while ensuring readability and type safety analysis. Each option is immediately understandable due to the clarity imparted by the dataclass framework.
Performance Considerations
Understanding the performance implications of Python dataclasses can illuminate their suitability for various programming contexts. In this section, we will analyze the key factors that affect performance, particularly in terms of memory usage and initialization speed. These aspects can influence decisions made when selecting the appropriateness of dataclasses for specific tasks, ultimately impacting the efficiency of an application.
Memory Usage Compared to Traditional Classes
When we use traditional classes in Python, we often define attributes explicitly within the method. Each instance created results in a memory footprint that can accumulate, potentially leading to inefficiencies, especially in applications that create numerous objects. In contrast, dataclasses optimize memory usage by utilizing a systematic approach to attribute management. Since dataclasses automate the creation of methods like , they help minimize the redundancy of specification, which often reduces memory overhead.
The key advantage here lies in their compact structure. Dataclasses store their attributes and aggregates metadata for efficiency.
A dataclass merely initializes memory for the attributes defined at the class level. This minimizes the script written and optimizes memory allocation significantly when large quantities of objects are involved.
A test evaluating memory consumption shows that the difference can be significant, especially when scaling up applications.
Speed of Initialization
In general, the initialization speed of an object correlates with how many attributes it manages and how efficiently the programmer can define behaviors. Dataclasses are designed to expedite the creation and setup of data attributes. Unlike traditional classes, where every instance sees attributes declared afresh, dataclass instances automatically inherit both attributes and methods from a structured base format.
As dataclasses leverage Python's built-in optimizations, the initialization thus benefits from a standard mechanism that creates attributes quicker than a manual setup. The automated generation of magical methods considerably reduces the human factor, re-structuring the process which aids in augmenting performance in critically timed applications.
Given specific benchmarks, using dataclasses is proven to streamline the workflow marked by lower initialization times. Developers and systems relying on systematic data structures discover they can maintain high-performance throughput efficiently while employing dataclasses as they fit neatly within the execution flow.
Best Practices for Using Dataclasses
Using dataclasses in Python can greatly simplify the task of handling data objects. However, understanding when and how to use them correctly is crucial. In this section, we will explore the best practices for implementing dataclasses effectively, ensuring they are not only functional but also maintainable and efficient.
When to Use Dataclasses
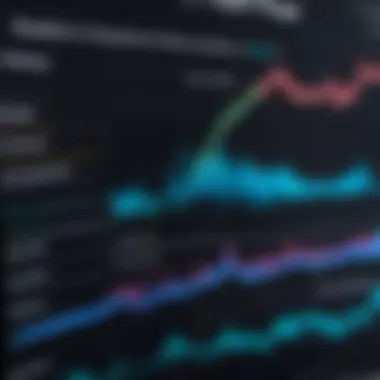
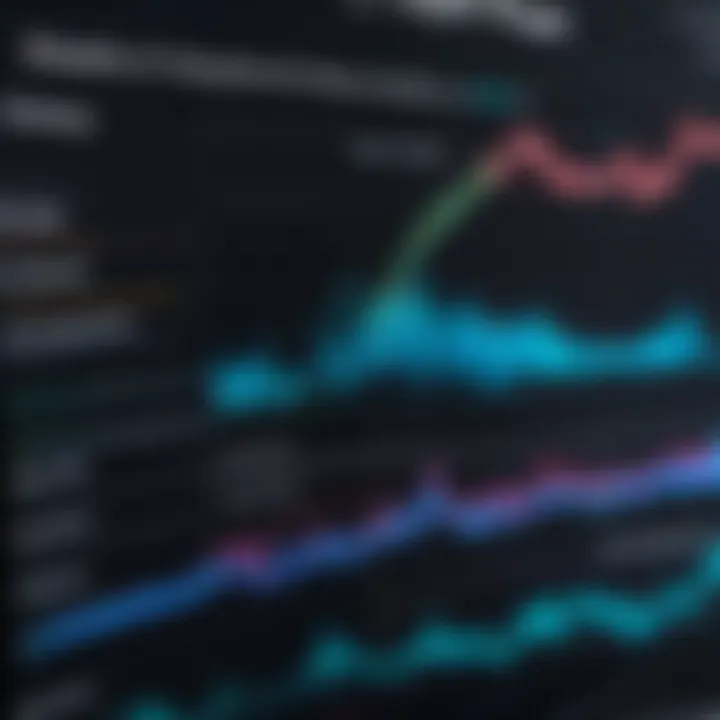
Dataclasses are particularly advantageous in scenarios where code maintenance and readability are a priority. The following conditions often make dataclasses a favorable choice:
- Storing and managing data: When building applications that involve intricate structures but center primarily on data storage, dataclasses are ideal. They remove much of the boilerplate code compared to traditional classes, enabling developers to focus on logic and data interpretation.
- Modeling structures: Dataclasses allow easier modeling of data structures such as configurations or entities that require type hints for precise conditions. This enhances both clarity and robustness in large projects.
- Sparse and simple data operations: If your project incorporates plain data handling — for instance, interfacing with web APIs or databases — dataclasses can minimize the required typing while maintaining functionality.
To illustrate, consider defining a simple data holder for a user profile:
In the above examples, you can see how straightforward using dataclasses can simplify your code. They automatically handle essential methods like , requiring only minimal boilerplate.
Avoiding Common Pitfalls
While the apparent simplicity of dataclasses can be alluring, they also present challenges that one must navigate carefully. Here are some features and usage habits to avoid misunderstandings and potential errors:
- Excesive Mutability: While immutability is an optional feature, excessive modification of dataclass instances leads to unintended side effects and debugging challenges. Use to ensure objects remain immutable. This can help forms a clearer contract within your codebase.
- Ignoring type hints: Utilizing type hints is one of the key benefits of dataclasses, enhancing code clarity and making it self-documenting. Omitting type hints can cause confusion, particularly for flower embedding and frameworks that rely on them for validation.
- Inconsistent field default values: When defining fields, it is essential to have a consistent initialization pattern. This includes utilizing immutable default values, which can avoid s under certain conditions. Prefer using factory functions for mutable types.
Keeping dataclasses lean and purposeful will significantly improve program robustness and reduce maintenance costs.
By attentively applying these principles when using dataclasses, developers can eliminate frustrations and embrace the strengths of Python’s modern features.
Limitations of Dataclasses
Understanding the limitations of Python dataclasses is important for software developers. While dataclasses simplify class creation and provide clarity, certain restrictions warrant consideration. Developers must evaluate when to use dataclasses versus traditional classes to achieve desired results while remaining aware of potential issues.
Serialization Issues
Serialization refers to the process of converting an object into a format that can be easily stored or transmitted and later reconstructed. While dataclasses can be serialized, challenges arise, especially with default values involving mutable types. When a dataclass is instantiated, any mutable default value such as lists or dictionaries won't be serialized as expected.
For example, if a dataclass has a field with a default mutable argument:
In the example above, both instances share the same list reference. This might lead to unexpected behavior, often causing blind spots during development. Programmers should consider using default factory functions for fields that need mutable default values. This design prevents shared references and unintended data modifications.
Complex Inheritance Scenarios
Dataclasses work well for straightforward use cases, but their handling of inheritance presents certain challenges. When subclasses are used, especially with multiple inheritance, complications may arise as Python's method resolution order (MRO) can yield unexpected results. Dataclasses don't always automatically prioritize their special methods. Thus, careful design in inheritance hierarchies is required to avoid ambiguity.
Consider a situation involving multiple base classes where method overrides or attribute definitions lead to conflicts. Dataclasses may inadvertently rely on fields from parent classes, leading to instances without clearly defined behavior.
The key aspect to keep in mind is: while dataclasses contribute to cleaner syntax, they can introduce complications in complex hierarchies. Use clear and unambiguous patterns when designing with dataclasses in an inheritance structure.
The End
In this article, the discussion of Python dataclasses has illustrated their growing significance in today’s programming landscape. Understanding dataclasses is crucial as they represent a keystone innovation that simplifies data-centric programming. Programmers gain a streamlined approach to create mutable and immutable data objects with less boilerplate code.
Summary of Key Points
Several key now fully highlighted core elements stand out from this articole on dataclasses:
- Definition: Dataclasses provide an easy way to define classes for holding data without requiring extensive setup.
- Special Methods: They automatically generate essential methods, which minimizes human error in implementations while following best practices.
- Default Values: The syntax allows easy specification of default values along with factory methods, handling diverse data needs effectively.
- Performance: Compared to traditional classes, dataclasses offer better memory usage and efficient initialization.
Pointing out these facts shows how dataclasses simplify workflows and thus, contribute to better quality code in projects.
Final Thoughts on Dataclasses
Ultimately, this examination showcases that Python dataclasses are not just a trend for programmers; they serve significant advantages in improving code maintainability and readability. Are they suitable for every project? No, like any tool, there are limitations. Programmers need to assess their specific use case regarding complexities, particularly in inheritance structures or serializing instances.
Keeping these considerations in mind allows a harmonious integration of dataclasses into existing codebases. This understanding spells the difference between simply using cods and using it effectively as part of an overall strategy to promote clean and maintainable codes.
As we move forward, recognizing situations for dataclasses could make a marked impact on the success of various software endeavors.
"Having clear and minimalistic code is not just an aim; it's a necessity that affects the scalability and dependability of projects."