Mastering Tree Traversal Algorithms: A Comprehensive Guide
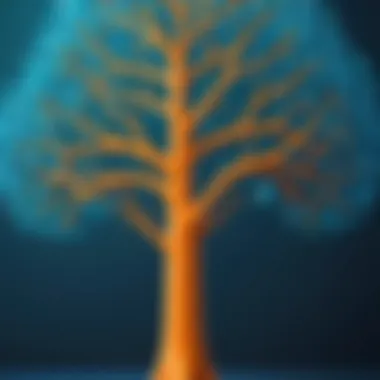
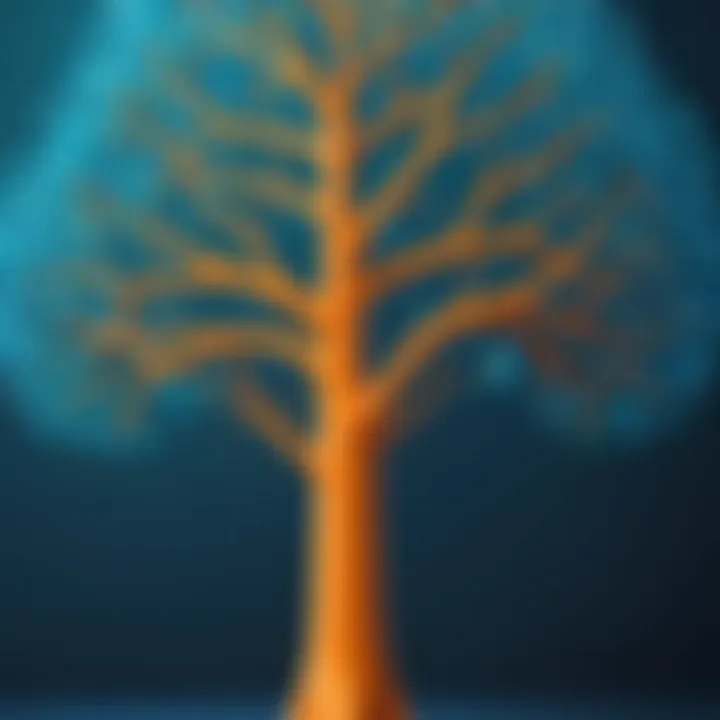
Intro
Tree traversal algorithms are fundamental to understanding how trees operate in computer science. A tree data structure provides an efficient way to organize and manage hierarchical data, allowing quick access to its elements. This article will inspect the primary traversal methods, namely, pre-order, in-order, and post-order. By outlining specifics related to algorithms and implementations, this resource aims to build a strong foundation for further exploration in tree-related coding challenges and technology trends.
Understanding tree traversal not only aids in grasping how data structures function but also impacts numerous domains in programming, including artificial intelligence, machine learning, and data management.
Drill down into the nitty-gritty of tree traversal techniques can arm tech enthusiasts with the skills to tackle complex and intricate problems that come up in software development.
Coding Challenges
Coding challenges often necessitate familiarity with how data structures work. Tree traversal is an important self-defining aspect of these structures.
Weekly Coding Challenges
Programming weekly challenges provide excellent practice for honing tree traversal skills. More platforms like LeetCode and HackerRank offer problems that involve traversing trees and numerous cases related to different traversal techniques.
Problem Solutions and Explanations
When solving problems, one must apply the correct traversal technique to reach the goal. For example, a common task could include traversing a binary tree to find a particular value. Different strategies lead to varied efficiency.
Tips and Strategies for Coding Challenges
- Plan Thoroughly: Before jumping into the coding part, outline the approach. Consider how the algorithm will interact with the tree structure.
- Keywords Knowledge: Ensure a solid understanding of the definitions of each traversal type instead of solely focusing on coding them.
- Test Officially: Use sample problems to validate code correctness. No code is complete until tested.
Community Participation Highlights
Communities like Reddit have forums dedicated to coding challenges where users can share solutions and insights. Engaging with like-minded individuals can foster learning and motivate improvement.
Technology Trends
Tree traversal algorithms fit into the broader scope of technology trends with rapidly-evolving work environments. Many organizations require understanding various data structures like trees to advance operations and enhance productivity.
Latest Technological Innovations
Emerging technologies efficiently utilize tree structures to deal with massive data retrieval processes. Trees are integral in databases and network systems as they significantly reduce access times.
Emerging Technologies to Watch
Stacked with fast algorithms and implementations, tree-based approaches impact the fields of search engine optimization and data analysis. Modeling data in trees is an invaluable starting point as technologies continue shifting to accommodate larger datasets.
Understanding tree traversal is key to advanced computational techniques.
Technology Impact on Society
The performance of sorting algorithms can determine access and retrieval efficiency across platforms crucial for user interaction. Think about artificial intelligence; efficient traversal algorithms directly affect the speed in learning models.
Expert Opinions and Analysis
Professionals are expressing rising demand for programmers proficient in data structures. The prowess in tree manipulation can set talented individuals apart in rigorous industries focused on software precision.
End
As seen, tree traversal algorithms not only provide a route through school curricula for computer science students, they forge an unequaled relevance in application development. Role of these algorithms cannot be understated in a world that steadily looks towards advanced data processing systems. A thorough grasp and practical experience in tree traversals prove to be valuable in expanding one’s technical repertoire.
Prologue to Tree Data Structures
Tree data structures serve as a fundamental concept in computer science, representing hierarchical data. This introductory section forms the basis for understanding more complex algorithms, specifically tree traversal algorithms. Having a solid grasp of tree structures enhances the reader's ability to navigate through these algorithms efficiently and effectively.
Definition of Tree Structures
A tree structure consist of nodes connected by edges. The model typically includes a single root node from which other nodes derive, forming a parent-child relationship. Each node can have multiple children, and the connections dictate the hierarchy. Here are some key elements:
- Root Node: The top node of the tree, with no parent.
- Branch: The edge connecting any two nodes.
- Leaf Node: A node without any children.
- Subtree: A smaller tree consisting of a node and its descendants.
The non-linear organization is especially useful in applications like databases, where it helps represent structured records and allows for efficient data retrieval.
Importance of Tree Structures in Computing
Tree structures have significant importance in various fields related to computing. One main advantage is their ability to facilitate efficient searching, inserting, and deletion operations, particularly with binary trees. For example, operations on balanced binary search trees, such as AVL or Red-Black trees, have time complexities of O(log n). This efficiency dramatically impacts data handling capabilities in larger and more complex systems.
Moreover, tree structures are employed in numerous practical applications. Examples include:
- File Systems: Organizing files in a hierarchical manner.
- Databases: Quick retrieval through indexing methods like B-trees.
- Artificial Intelligence: Representing decision-making trees.
Understanding tree structures also lays the groundwork for analysis. Any applicable algorithm that processes tree structures often relies on these definitions. As infer branches out further into various traversal algorithms, a solid foundation helps absorb subsequent themes effectively.
In summary, grasping tree structures is vital for comprehending the algorithms built upon them. This knowledge promotes improved programming practices and enhances algorithmic thinking.
What is Tree Traversal?
Tree traversal is a crucial aspect of working with tree data structures. It defines the process of visiting all the nodes in a tree, allowing for operations like searching, sorting, and updating data. Traversing a tree provides insight into its structure, organization, and the interrelations between elements. This article intends to illuminate the concept of tree traversal and its diverse applications, making it accessible for aspiring and experienced programmers alike.
Understanding Traversal Processes
Traversing a tree involves systematic approaches to reach every node, ensuring no segment is overlooked. The methods of tree traversal can be broadly grouped into two categories: depth-first traversal and breadth-first traversal.
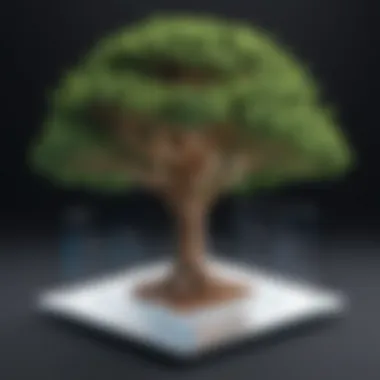
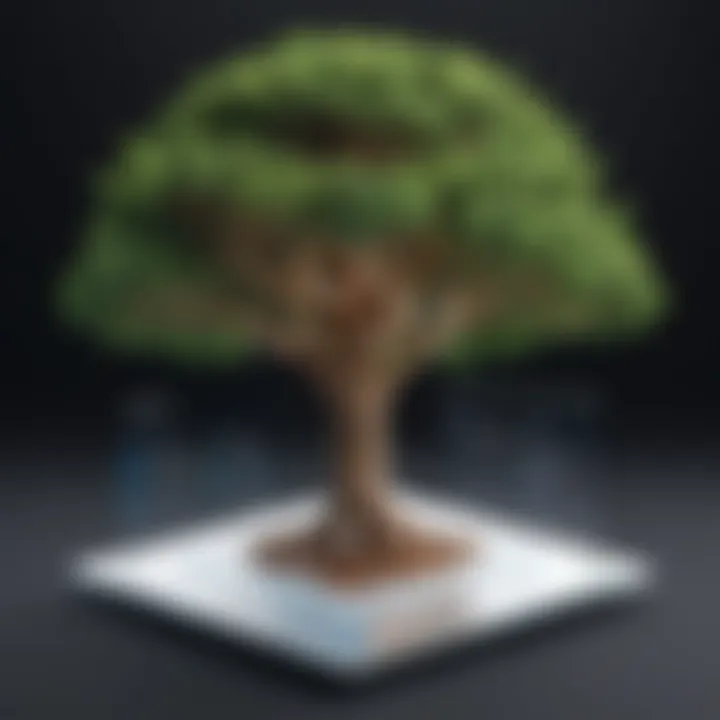
In depth-first traversal, the algorithm prioritizes the depth of a branch. The traversal focuses on exploring as far down a branch as possible before backtracking. This can further be categorized into three methods: pre-order, in-order, and post-order, each determining the sequence in which nodes are processed.
On the other hand, breadth-first traversal examines nodes layer by layer, level by level, beginning with the root and moving horizontally across each subsequent layer.
Implementing these processes effectively enhances programming practices. By knowing when and how to apply each traversal method, developers can optimize algorithm performance, resulting in improved efficiency and reduced computational cost.
Categories of Traversal Methods
Each traversal method serves specific purposes. Understanding these categories assists in selecting the correct approach for the right scenario.
- Depth-First Traversal:
- Breadth-First Traversal:
- Pre-order Traversal: Visits the root node first, followed by the left subtree, and then the right subtree. Suitable for scenarios where the parent node's information is necessary before exploring the child nodes.
- In-order Traversal: Visits the left subtree first, then the root node, and finally the right subtree. This method is effective for producing sorted output when dealing with binary search trees.
- Post-order Traversal: Visits the left and right subtrees before processing the root node. Helpful in scenarios requiring the completion of child node operations prior to addressing the parent.
- Level-Order Traversal: Visits nodes layer by layer. This method is particularly useful for applications such as shortest path finding in graphical representations or implementing queue structures.
Understanding these traversal categories significantly influences algorithm design. Each method's characteristics and performance implications should be carefully considered. Ultimately, the choice of a traversal technique is informed by the data's nature and the intended operations.
Types of Tree Traversal Algorithms
Tree traversal algorithms are fundamental to the functioning of data structures and their optimization in algorithms, especially in computer science. These algorithms outline how a tree can be systematically visited. Understanding these traversal types enables programmers to select the best method for specific situations, balancing efficiency and clarity for a range of applications.
When implementing tree data structures, the concept of traversal takes root as a way to explore nodes systematically. Not only does this practice reveal the structure, but it also has implications for data retrieval, manipulation, and overall performance.
Depth-First Traversal
Depth-First Traversal (DFT) techniques delve into the depth of a node progressively before visiting sibling nodes. This approach emphasizes a pathway from the root to the deepest leaves.
Pre-order Traversal
In Pre-order Traversal, the key characteristic is that the root node is processed first before its child nodes. Typically, a structure is printed or acted upon before visiting its subtrees. This facilitates operations where the direct hierarchy is meaningful, such as when constructing a copy of the tree.
This traversal performs the following steps:
- Visit the root node
- Recursively do a pre-order traversal of the left subtree
- Recursively do a pre-order traversal of the right subtree
Pre-order Traversal shines in scenarios demanding prefix notation and generating or validating nested data structures. Ahead of others, it is favored for ease of implementation, yet care must be taken with performance in deep or large trees where stack overflow is a potential issue.
In-order Traversal
In-order Traversal stands out as a method that naturally sorts data. The key aspect of this traversal is visiting the left subtree first, then processing the root, and finally the right subtree. As a benefit, In-order traversals on a Binary Search Tree yield sorted values.
This technique follows these steps:
- Recursively do an in-order traversal of the left subtree
- Visit the root node
- Recursively do an in-order traversal of the right subtree
In locations requiring sorted access, In-order possesses unmatched advantages. However, it may not yield straightforward outcomes outside trees explicitly designed for such traversal,
Post-order Traversal
Post-order Traversal emphasizes completeness; the child nodes are fully explored before accessing their parent node. The key characteristic shows it as advantageous for deletion processes and organizing cleanup tasks in trees.
The following actions are executed:
- Recursively do a post-order traversal of the left subtree
- Recursively do a post-order traversal of the right subtree
- Visit the root node
Its unique advantage lies in offering a precise means for tasks needing post-navigational actions, such as memory efficiency or structured data removal. Users must be mindful that larger trees delay results compared to other traversal methods.
Breadth-First Traversal
In contrast, Breadth-First Traversal (BFT) explores the nodes level by level from the root. Its processing method advocates for thorough visibility across the tree on each level before descending further.
Level-Order Traversal
Level-Order Traversal epitomizes the BFT method, ensuring nodes at the same depth are visited sequentially. This key characteristic makes it suitable for search operations or when spatial data relationship assessments are crucial.
This traversal type utilizes a queue and executes:
- Dequeue the front node
- Process it and enqueue the left and right children
Level-Order contributes positively to scenarios like scheduling and graph mapping. Its structured visits appeal for visual representations, though it may consume more memory compared to depth-first approaches due to storing interim data points.
In reviewing tree traversal algorithms, selecting a method hinges upon the desired structure and outcome, underlining the abundant utility within software and database management.
Pre-order Traversal
Pre-order traversal is a crucial concept in the study of tree algorithms. This traversal method is unique in its approach, allowing programmers to access processes in a very specific sequence. Understanding pre-order traversal lays the groundwork for appreciating other traversal techniques as well. Its unique attributes and applications in computer science necessitate a thoughtful exploration.
Algorithm Explanation
The algorithm for pre-order traversal can be described succinctly. It follows three steps: first, visit the root node, then traverse the left subtree in pre-order, and finally, traverse the right subtree in pre-order. The fundamental structure of the algorithm can be implemented recursively, giving it internal clarity. Below is the simplified formulation of the pre-order traversal algorithm:
- Visit Node
- Traverse Left Subtree
- Traverse Right Subtree
This method guarantees that each node will be visited before any of its child nodes.
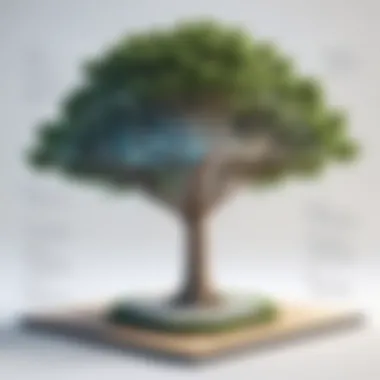
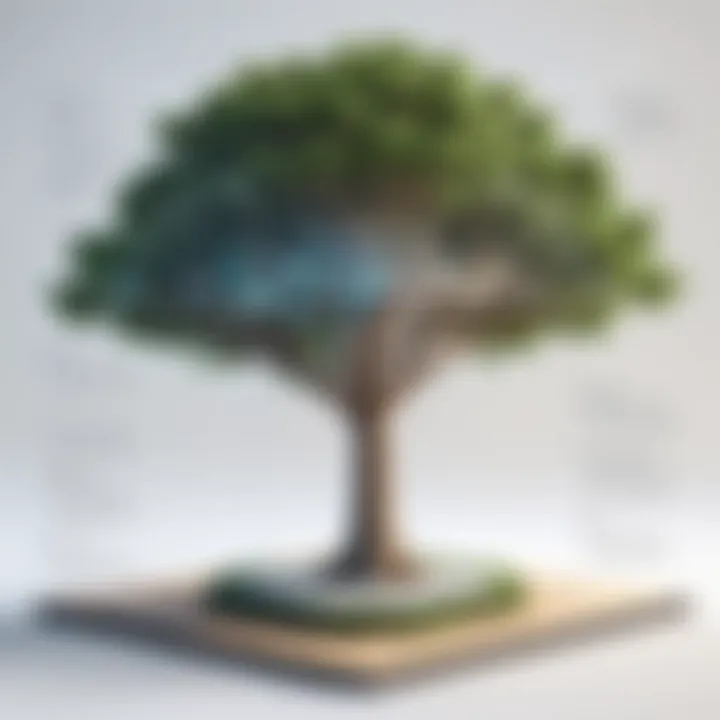
Implementation Example
Let's look at pre-order traversal in the context of a binary tree. Below is a concise piece of code for implementation, using pseudo-code to keep it clear, yet easy to follow:
In this example, we define the function , which takes a node as an input. The base case checks for a null node, and if the node exists, it's processed before descending to its children. This neatly encapsulates the essence of pre-order traversal as elaborated previously.
Use Cases for Pre-order Traversal
Pre-order traversal is not just an abstract concept. Its utility spans various practical scenarios in computer science. Here are several key applications:
- Generating Prefix Expressions: In compilers, pre-order traversal enables the creation of prefix notation (also known as Polish notation). This method simplifies expression evaluation.
- Cloning Trees: When duplicating tree structures, traversing in pre-order guarantees a comprehensive replication of the components from the root downward.
- Hierarchy Representation: This traversal helps represent classes or categories in order, particularly beneficial in organizational charts or JSON object traversal in JavaScript frameworks.
Pre-order traversal, while simple in its approach, holds immense practicality and relevance across numerous computer science disciplines.
In-order Traversal
In-order traversal is a key algorithm for tree data structures. It is specifically significant because it systematically processes the nodes of a binary tree and retrieves the values in a logically ascending order. This property makes in-order traversal particularly valuable in cases where sorted data is essential, such as generating output for binary search trees where nodes follow specific ordering rules.
By employing in-order traversal, one can examine all nodes in the left subtree, then simply access the parent node, followed by nodes in the right subtree. The method guarantees that users can effortlessly retrieve values in a structured manner. Therefore, the algorithm's design inherently supports tasks that require an orderly examination of tree data.
Algorithm Explanation
The in-order traversal algorithm can be detailed as follows. It involves a recursion-like approach that navigates through binary trees. Starting at the root node, this algorithm first traverses to the left node recursively. Once it reaches the leftmost node, it begins recording those values.
After fully processing the left subtree, it accesses the current node and records its value. After that, it proceeds recursively to the right node. This video describes tree navigation of in-order tree traversal:
- Start at the root.
- If the left child exists, call in-order on it.
- Visit the node (save value).
- If the right child exists, call in-order on it.
The tree visualization during traversal might resemble:
In this example, inorder traversal yields the sequence: D, B, E, A, C, F.
Implementation Example
To grasp the concept of in-order traversal thoroughly, let's look at a simple implementation in Python:
In this snippet, the function calls itself recursively moves through the tree nodes. It prints the node values in the correct order, demonstrating how the algorithm works seamlessly.
Applications of In-order Traversal
In-order traversal finds promising ground in various applications, showcasing its utility in real-world scenarios. Below are some notable applications:
- Binary Search Trees: In-order traversal effectively retrieves values in a sorted manner. This property is crucial when the end-users expect organized data output, often needed for databases and data management applications.
- Expression Tree Evaluations: In creating parsers and evaluators for arithmetic expressions, in-order traversal aids in generating proper notation. Operators and operands can amalgamate neatly by following this approach.
- Database Systems: The method enables efficient querying as it uses sorted structure mobility, considerably enhancing performance in data retrieval processes.
Utilizing this traversal technique allows optimal interaction with the data models, contributing to increased efficacy in programming contexts.
Post-order Traversal
Post-order traversal is a critical part of the family of tree traversal algorithms. This method focuses on visiting all the nodes of a tree in a specific sequence. The sequence for post-order is unique: it traverses the left subtree, then the right subtree, and finally the node itself. This might seem counterintuitive at first, but understanding its practical applications will highlight its significance.
The importance of post-order traversal lies in its practical applications, especially in scenarios requiring operations on a tree that involve both processing of child nodes before their parent nodes and memory management tasks.
Algorithm Explanation
When describing post-order traversal algorithmically, we are implementing a systematic approach. The algorithm can be outlined as follows:
- If the current node is not null, proceed with the following steps:
- Recursively call post-order traversal on the left child.
- Recursively call post-order traversal on the right child.
- Visit the current node.
This approach ensures that every child node is processed before its parent. The following pseudo code demonstrates this:
The operation is recursive in nature. This efficiency is a primary factor in its effectiveness, making post-order traversal a fundamental concept in the computer science field.
Implementation Example
To provide clarity on how post-order traversal works in practice. Let’s assume we have the following tree:
To implement post-order traversal, we can structure our visit results:
- Starting with A, we traverse to B.
- From B, we move to D and visit D first.
- We continue to E and visit E next.
- Return to B and that's visit B.
- Next, we turn to C and go to F, visiting F after.
- Finally, we visit C and finish with A.
The traversal result is: D, E, B, F, C, A. Such an organized approach clearly highlights how children are addressed before their parents, reinforcing the algorithm's integrity.
Use Cases for Post-order Traversal
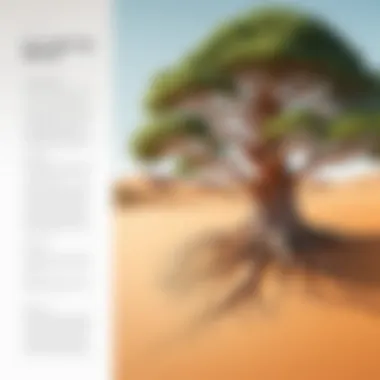
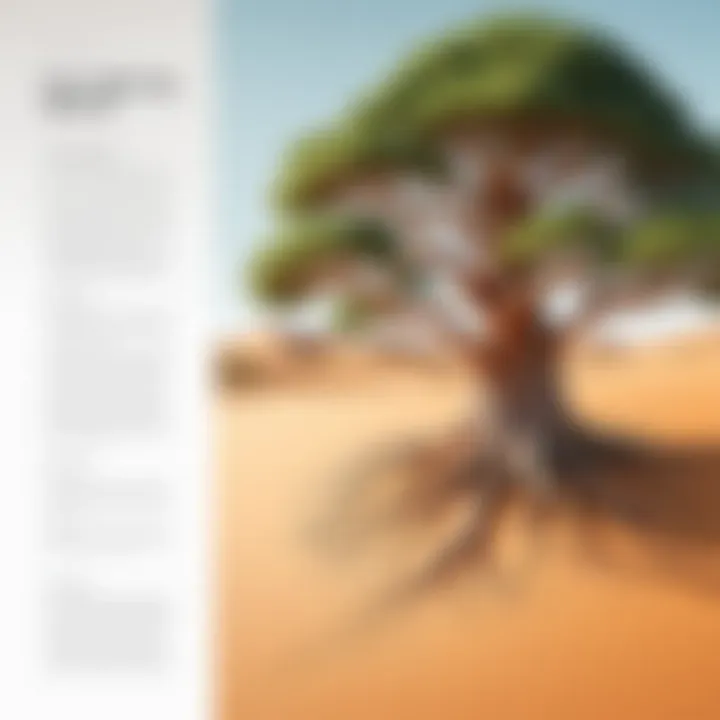
Post-order traversal finds its niche in specific programming scenarios. Understanding its use cases enhances practical development skills. The most prominent use cases include:
- Tree destruction: Helps in correctly deallocating memory used by tree nodes. By visiting children first, a program ensures that the tree is deleted in a systematic manner.
- Expression evaluation: Useful in processing expression trees in data management applications. The result relies on the right precautions: processing operands before applying operators.
- File directories: Supports filesystem navigation by addressing files after addressing the directory component. This ensures efficient retrieval of data from complex structures.
By leveraging post-order traversal, programmers foster noteworthy efficiencies in these applications, thus reflecting on its undeniable relevance in contemporary programming.
Post-order traversal ensures that child processes complete before any parent node begins its operations.
Breadth-First Traversal
Breadth-First Traversal, often referred to as Level-Order Traversal, is a crucial method in the study of tree data structures. Its significance resides in its approach, where nodes are explored level by level, moving horizontally across the tree. This contrasts sharply with Depth-First methods that go deep down into branches before exploring siblings. The systematic exploration of levels in breadth-first traversal enables a variety of applications where the layer of data is pertinent, such as in network broadcasting and pathfinding algorithms.
Algorithm Explanation
The core concept behind Breadth-First Traversal is simple yet powerful. This method utilizes a queue data structure to track nodes for exploration. Starting from the root node, it places its immediate children into the queue. The process repeats as follows:
- Remove the front node from the queue.
- Visit the node (i.e., process it or record its value).
- Add all its children to the back of the queue.
- Continue until the queue is empty.
In this algorithm, it linearizes the hierarchical structure in a manageble order that prioritizes levels over depth.
Implementation Example
To demonstrate Breadth-First Traversal, consider a standard implementation with a simple tree structure. Suppose we have this tree:
Using a Breadth-First approach, we would explore the nodes in this sequence: A, B, C, D, E, F, G. Below is an implementation snippet in Python:
This concise code effectively facilitates breadth-first exploration, showcasing how this method traverses the entire network of nodes efficiently.
Applications of Level-Order Traversal
Breadth-First Traversal finds its utility across various technical realms. For instance, it is widely employed in network routing algorithms to find the shortest path between nodes. It can also analyze the social networking graphs in applications like Facebook. Here are additional applications:
- Scene representation in video games to create detailed maps.
- Communication broadcasts where every node needs to receive packets simultaneously.
- Solving puzzles such as the shortest path to solve a grid-like maze.
Traversal Algorithm Comparisons
Traversal algorithm comparisons are critical for programmers and computer scientists. Choosing the right traversal method can significantly influence the performance of a program. Considering the unique strengths and weaknesses of each method will ensure that the right tool is selected for particular scenarios. This section will discuss efficiency and complexity analysis along with how to choose the appropriate traversal method.
Efficiency and Complexity Analysis
When comparing traversal algorithms, understanding their efficiency and complexity is essential. Efficiency often refers to both time and space complexity.
- Time Complexity: This metric investigates how the algorithm performance scales with respect to input size. Each traversal method, such as depth-first and breadth-first, has different time complexities.
- Depth-First Search (DFS): Generally operates in O(V + E) time, where V is the number of nodes and E is the number of edges.
- Breadth-First Search (BFS): Also O(V + E) but has a different approach to traversal.
- Space Complexity: This deals with the memory usage of the algorithm. Space requirements can become critical, especially with large data sets.
- DFS: The worst-case space complexity is O(h), where h is the height of the tree. In scenarios with skewed trees, it can degrade significantly.
- BFS: Here, the space complexity can rise to O(w), where w is the maximum width of the tree at any level.
This comparison between time and space complexity helps in selecting which traversal technique may be more effective based on use cases.
Understanding these complexities not only optimizes performance but also enables advancements in application design.
Choosing the Right Traversal Method
Selecting the correct traversal method depends on multiple factors. Here are some elements to consider:
- Nature of Data: Depending on if data is more tree-like or graph-like.
- Use Case Requirements: Some tasks may require all nodes to be visited, while others may only need parts of them.
- Performance Considerations: The analysis from the previous section offers clear guidelines. Using algorithms like depth-first for solutions with fewer resources can make handling smaller data structures far easier.
Decision Framework
Here's a simplified framework to assist in decision-making:
- For Complete Exploration of Data: Use DFS. It’s optimal where memory usage needs to be minimized and depth needs to be prioritized.
- For Shortest Path Problems in Graphs: Consider BFS, as it explores layer by layer, making finding the shortest path straightforward.
- For Persistence Needs: Depending on how deep or wide the tree will behave, selecting BFS can avoid pitfalls with large memory consumption.
Closure on Tree Traversal Algorithms
Tree traversal algorithms are fundamental to the understanding and development of tree data structures. The methods of traversal we have discussed—pre-order, in-order, post-order, and breadth-first—serve not only as essential techniques in programming but also highlight various approaches to navigating the complexity of tree structures efficiently.
Each traversal method has its unique algorithms, implementations and real-world applications, making them invaluable in computer science. In this article, we explored the distinctions among these methods and how they hold up against various efficiency metrics. This highlights that the choice of a traversal method impacts performance within tree-based applications.
Summary of Key Points
- Traversal Methods: We examined four primary methods, recognizing how each supports specific use cases.
- Algorithm Understanding: A grasp of traversal algorithms allows programmers to manipulate data within trees effectively, enabling optimized performance.
- Efficiency Complexity: Each method offers different efficiencies, influencing system resource usage and algorithm performance.
- Practical Applications: The relevance of these methods spans areas such as database operations, memory management, and AI structure decision trees.
The choice of a tree traversal algorithm significantly impacts both computational efficiency and the complexity of binary search tasks.
Future Directions in Tree Traversal Research
As technology advances, tree traversal techniques will likely evolve as well. Research may focus on several areas:
- Optimizing Existing Algorithms: Continued work is expected to refine existing algorithms for better time and space complexity.
- Dynamic Structures Adaptations: As dynamic and real-time data structures gain prominence, traversal methods will need adjustment and reinforcement to manage diverse datasets in these frameworks.
- Application of AI: Integrating AI methodologies into tree traversal could revolutionize problem-solving, making automated these choices based on vast datasets.
- Distributed Systems Focus: Exploring improved strategies for tree traversal in distributed architectures will likely enhance performance in cloud computing scenarios.
This article serves as a foundation for anyone delving deeper into tree traversal algorithms. Understanding these concepts will equip aspiring programmers and experienced IT professionals to make informed decisions and foster further inquiries into this essential area.